一、FileReader和FileWriter的使用
1、数据读入操作
说明:
①read():返回读入的第一个字符,当读到文档末尾,返回-1
②异常的处理:为了保证流资源一定会执行关闭操作,要对异常进行try-catch-finally处理
③对于读入操作,指定的文件一定要存在,否则会抛FileNotFoundException异常
/*
读取day09下的hello.txt文件,并将文件中的内容输出到控制台
说明:
1.read():返回读入的第一个字符,当读到文档末尾,返回-1
2.异常的处理:为了保证流资源一定会执行关闭操作,要对异常进行try-catch-finally处理
3.对于读入操作,指定的文件一定要存在,否则会抛FileNotFoundException异常
*/
@Test
public void test1FileReader1(){
FileReader fr = null;
try {
//1.提供指定的File对象
File file1 = new File("hello.txt");
//2.提供流的对象
fr = new FileReader(file1);
//3.数据的操作
char[] cbuf = new char[5];
int len;
while((len = fr.read(cbuf)) != -1){
//方式一:
//错误的写法
// for (int i = 0; i < cbuf.length; i++) {
// System.out.print(cbuf[i]);
// }
//正确的写法
// for (int i = 0; i < len; i++) {
// System.out.print(cbuf[i]);
// }
//方式二:
//错误的写法:对应方式一错误的写法
// String str = new String(cbuf);
// System.out.print(str);
//正确的写法:对应方式一正确的写法
String str = new String(cbuf,0,len);
System.out.print(str);
}
} catch (IOException e) {
throw new RuntimeException(e);
} finally {
if(fr != null){
//4.关闭流
try {
fr.close();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
}
}
2、数据写出操作
说明
①输出操作指明的文件可以不存在。并不会抛出异常。
②如果指明的文件在硬盘中不存在,在写出数据过程中,会自动生成相应的文件。
如果指明的文件在硬盘中已存在:
且相对应的FileWriter流调用的构造器是:FileWriter(file,false)或FileWriter(file):会对原有的文件进行覆盖。
相对应的FileWriter流调用的构造器是:FileWriter(file,true):不会对原有文件进行覆盖,只会对原有文件的基础上追加内容。
/*
从内存中写出数据到硬盘的指定文件
说明
1.输出操作指明的文件可以不存在。并不会抛出异常。
2.如果指明的文件在硬盘中不存在,在写出数据过程中,会自动生成相应的文件。
如果指明的文件在硬盘中已存在:
且相对应的FileWriter流调用的构造器是:FileWriter(file,false)或FileWriter(file):会对原有的文件进行覆盖。
相对应的FileWriter流调用的构造器是:FileWriter(file,true):不会对原有文件进行覆盖,只会对原有文件的基础上追加内容。
*/
@Test
public void testFileWrite() throws IOException {
//1.提供写出数据到的文件
File file = new File("hello1.txt");
//2.提供FileWrite流
FileWriter fw = new FileWriter(file,false);
//3.写出操作
fw.write("I have a dream!\n");
fw.write("You need to have a dream!");
//4.关闭流
fw.close();
}
3、综合输入输出流:复制粘贴操作
/*
从内存读入指定数据并写出到指定文件:复制粘贴操作
*/
@Test
public void testFileReaderFileWrite(){
FileReader fr = null;
FileWriter fw = null;
try {
//1.提供File类的对象
File file1 = new File("hello.txt");
File file2 = new File("hello2.txt");
//不能使用字符流处理图片等字节文件
// File file1 = new File("绿地.jpeg");
// File file2 = new File("绿地1.jpeg");
//2.提供输入、输出流
fr = new FileReader(file1);
fw = new FileWriter(file2);
//3.读入、写出操作
char[] cbuf = new char[5];
int len;
while((len = fr.read(cbuf)) != -1){
fw.write(cbuf,0,len);
}
} catch (IOException e) {
throw new RuntimeException(e);
} finally {
//4.关闭流资源
//方式一:
// if(fw != null) {
// try {
// fw.close();
// } catch (IOException e) {
// throw new RuntimeException(e);
// }finally {
// if(fr != null) {
// try {
// fr.close();
// } catch (IOException e) {
// throw new RuntimeException(e);
// }
// }
// }
// }
//方式二
if(fw != null){
try {
fw.close();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
if(fr != null){
try {
fr.close();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
}
}
二、FileInputStream和FileOutputStream的使用
1、数据读入操作
//使用字节流FileInputStream处理文本文件可能出现乱码
@Test
public void testFileInputStream(){
FileInputStream fis = null;
try {
//1.造文件
File file = new File("hello.txt");
//2.造流
fis = new FileInputStream(file);
//3.读入操作
byte[] cbuf = new byte[5];
int len;
while ((len = fis.read(cbuf)) != -1){
String str = new String(cbuf,0,len);
System.out.print(str);
}
} catch (IOException e) {
throw new RuntimeException(e);
} finally {
if(fis != null){
//4.关闭流
try {
fis.close();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
}
}
本地文件内容:

测试结果:

2、综合输入输出流:复制功能
/*
复制指定的图片
*/
@Test
public void testFileInputFileOutput(){
FileInputStream fis = null;
FileOutputStream fos = null;
try {
File srcFile = new File("绿地.jpeg");
File destFile = new File("绿地1.jpeg");
fis = new FileInputStream(srcFile);
fos = new FileOutputStream(destFile);
byte[] cbuf = new byte[5];
int len;
while((len = fis.read(cbuf)) != -1){
fos.write(cbuf,0,len);
}
} catch (IOException e) {
throw new RuntimeException(e);
} finally {
if(fos != null){
try {
fos.close();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
if(fis != null){
try {
fis.close();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
}
}
对于字节流FileInputStream和FileOutputStream的测试
结论:
1.处理文本文件(.txt、.c、.cpp、.java等类型的文件)应当使用字符流。
2.处理非文本文件(.jpg、.mp4、.avi、.doc、.ppt等类型的文件)应当使用字节流。
3、将复制操作封装为一个方法
/*
将复制操作封装为一个方法
*/
public void CopyFile(String srcPath,String destPath){
FileInputStream fis = null;
FileOutputStream fos = null;
try {
File srcFile = new File(srcPath);
File destFile = new File(destPath);
fis = new FileInputStream(srcFile);
fos = new FileOutputStream(destFile);
byte[] cbuf = new byte[1024];
int len;
while((len = fis.read(cbuf)) != -1){
fos.write(cbuf,0,len);
}
} catch (IOException e) {
throw new RuntimeException(e);
} finally {
if(fos != null){
try {
fos.close();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
if(fis != null){
try {
fis.close();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
}
}
测试:
@Test
public void testCopyFile(){
long start = System.currentTimeMillis();
String srcPath = "C:\\Users\\suxintai\\Desktop\\01-视频.mp4";
String destPath = "C:\\Users\\suxintai\\Desktop\\02-视频.mp4";
// String srcPath = "hello.txt";
// String destPath = "hello3.txt";
CopyFile(srcPath,destPath);
long end = System.currentTimeMillis();
System.out.println("复制视频花费的时间为" + (end-start));//605
}
测试结果:
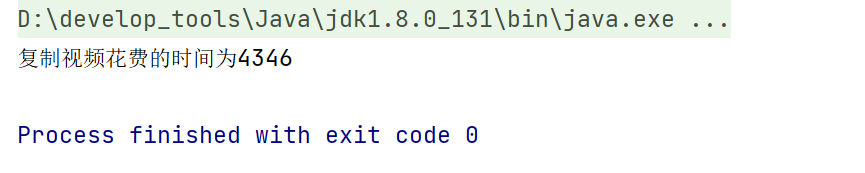
复制此视频花费4346毫秒。
复制的视频与原视频字节数相同。
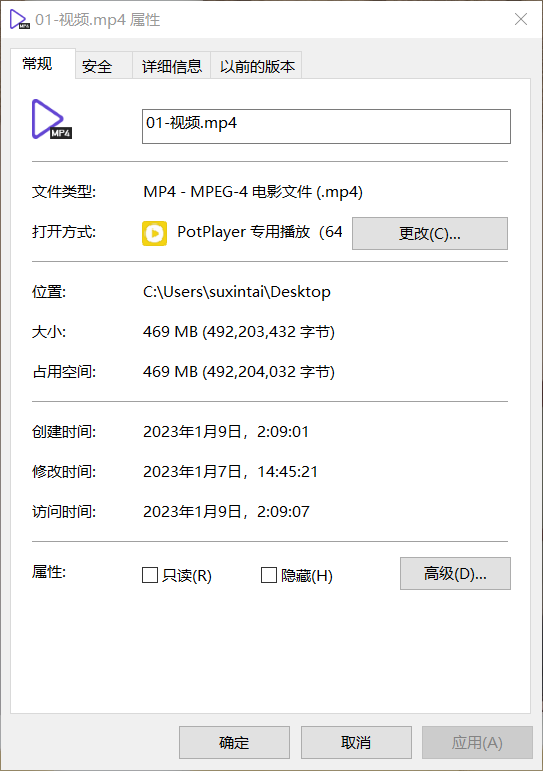
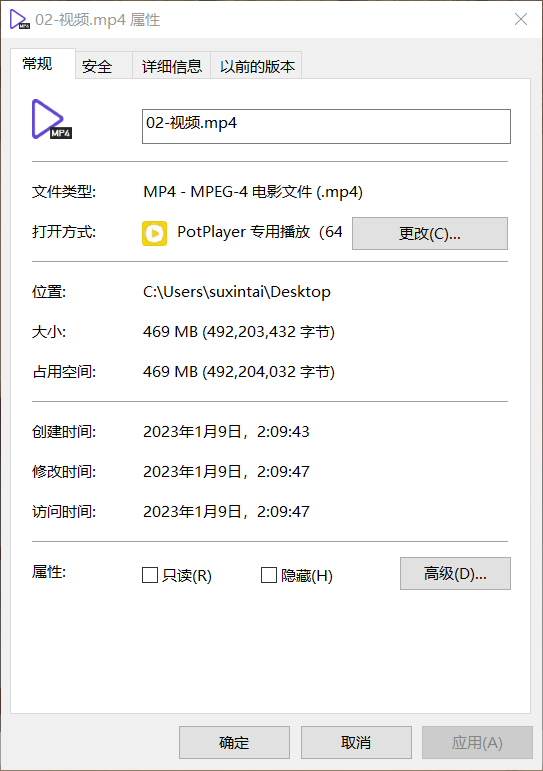