1.编写链表,链表里面随便搞点数据,使用 fprintf 将链表中所有的数据,保存到文件中,使用 fscanf 读取文件中的数据,写入链表中
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct ListNode {
int data;
struct ListNode* next;
};
// 链表操作函数
struct ListNode* createList() {
struct ListNode* head = NULL;
struct ListNode* curr = NULL;
// 插入节点到链表
int num;
printf("请输入链表数据(以 0 结束):\n");
scanf("%d", &num);
while (num!= 0) {
struct ListNode* newNode = (struct ListNode*)malloc(sizeof(struct ListNode));
newNode->data = num;
newNode->next = NULL;
if (head == NULL) {
head = newNode;
} else {
curr->next = newNode;
}
curr = newNode;
scanf("%d", &num);
}
return head;
}
// 将链表数据保存到文件
void saveListToFile(struct ListNode* head, const char* filename) {
FILE* file = fopen(filename, "w");
if (file == NULL) {
printf("无法打开文件!\n");
return;
}
struct ListNode* curr = head;
while (curr!= NULL) {
fprintf(file, "%d\n", curr->data);
curr = curr->next;
}
fclose(file);
printf("链表数据已成功保存到文件!\n");
}
// 从文件中读取数据并写入链表
struct ListNode* readListFromFile(const char* filename) {
FILE* file = fopen(filename, "r");
if (file == NULL) {
printf("无法打开文件!\n");
return NULL;
}
struct ListNode* head = NULL;
struct ListNode* curr = NULL;
int data;
// 从文件中读取数据并插入链表
while (fscanf(file, "%d", &data) == 1) {
struct ListNode* newNode = (struct ListNode*)malloc(sizeof(struct ListNode));
newNode->data = data;
newNode->next = NULL;
if (head == NULL) {
head = newNode;
} else {
curr->next = newNode;
}
curr = newNode;
}
fclose(file);
return head;
}
int main() {
struct ListNode* list = createList();
// 保存链表数据到文件
saveListToFile(list, "list_data.txt");
struct ListNode* newList = readListFromFile("list_data.txt");
// 遍历链表并打印数据
if (newList!= NULL) {
struct ListNode* curr = newList;
while (curr!= NULL) {
printf("%d ", curr->data);
curr = curr->next;
}
printf("\n");
} else {
printf("从文件中读取数据失败!\n");
}
return 0;
}
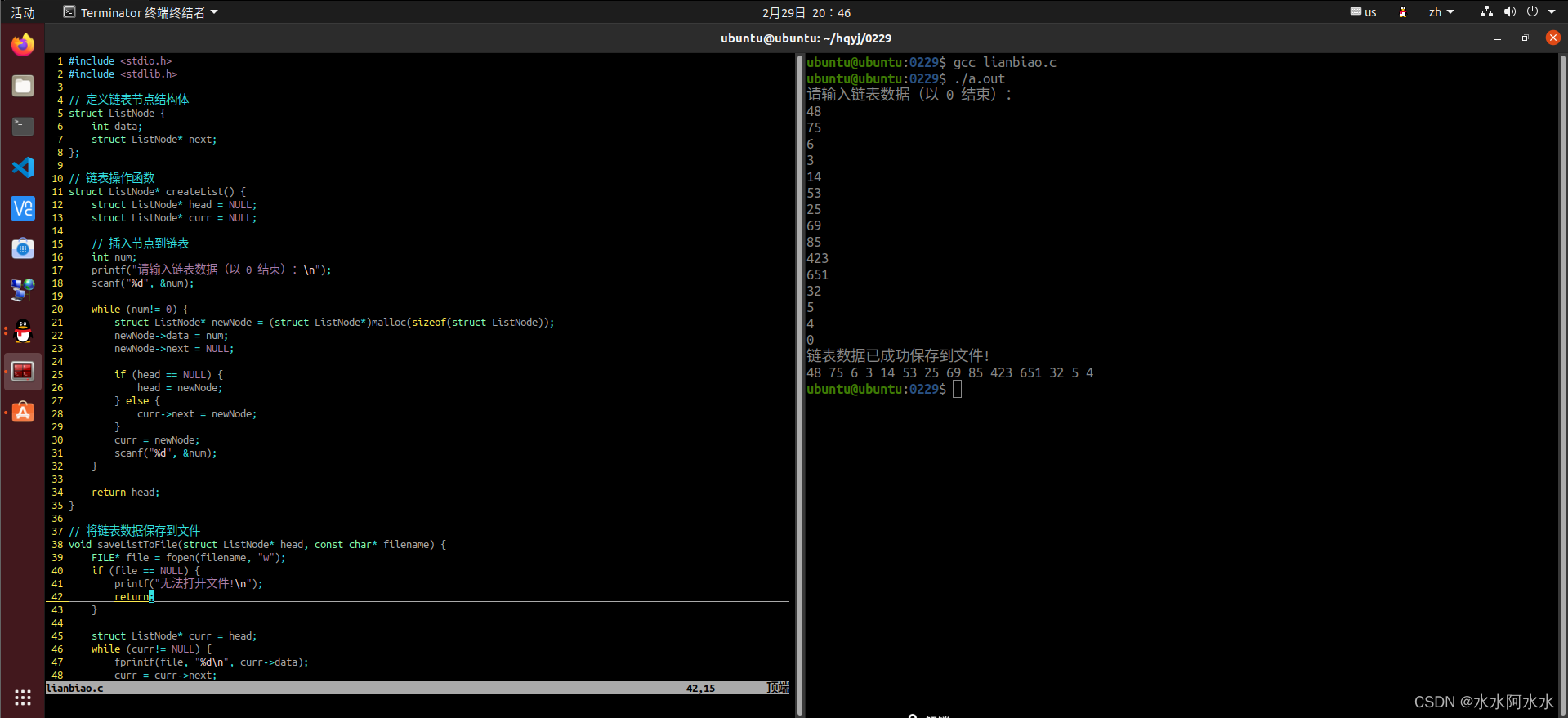