一.管道
管道是一种文件形式,是内核的一块缓冲区。匿名管道只能用于具有亲缘关系的进程间通信,命名管道可以用于任意的进程间通信。管道自带同步与互斥功能(读写操作数据大小不超过PIPE_BUF大小,读写操作受保护)。无名管道是一种内存文件,只能用于具有亲缘关系的进程间通信,fork的方式。管道是半双工的通信方式,数据只能单向流动,而且只能在具有亲缘关系的进程间使用。进程的亲缘关系通常是指父子进程关系。虽然管道是半双工的,但是我们可以通过创建两个无名管道实现父子进程间的相互通信。
(1)无名管道
1.pipe()函数
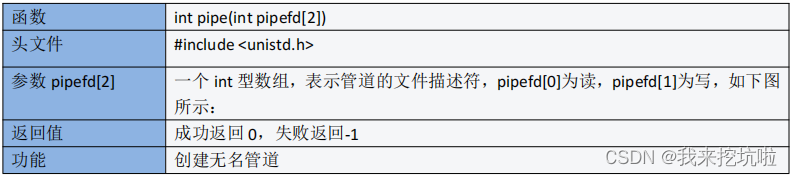
2.代码
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <sys/wait.h>
#include <sys/types.h>
#include <sys/stat.h>
int main(void)
{
char buf[32] = {0};
pid_t pid;
// 定义一个变量来保存文件描述符
// 因为一个读端,一个写端,所以数量为 2 个
int fd[2];
// 创建无名管道
pipe(fd);
printf("fd[0] is %d\n", fd[0]);
printf("fd[2] is %d\n", fd[1]);
// 创建进程
pid = fork();
if (pid < 0)
{
printf("error\n");
}
if (pid > 0)
{
int status;
close(fd[0]);
write(fd[1], "hello", 5);
close(fd[1]);
wait(&status);
exit(0);
}
if (pid == 0)
{
close(fd[1]);
read(fd[0], buf, 32);
printf("buf is %s\n", buf);
close(fd[0]);
exit(0);
}
return 0;
}
3.结果
(2)有名管道
1.mkfifo()函数
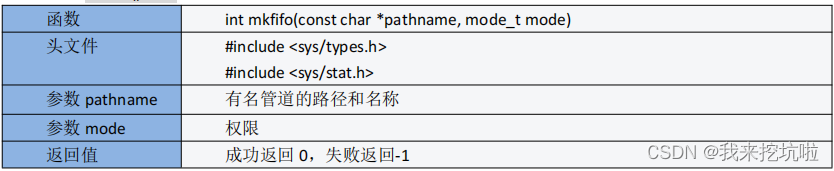
2.使用步骤
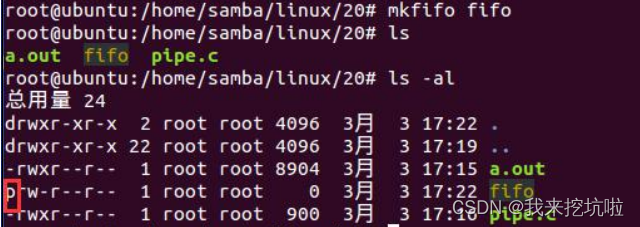
3.实验代码
#include <unistd.h>
#include <stdlib.h>
#include <sys/wait.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
int main(int argc, char *argv[])
{
int ret;
char buf[32] = {0};
int fd;
if (argc < 2)
{
printf("Usage:%s <fifo name> \n", argv[0]);
return -1;
}
if (access(argv[1], F_OK) == 1)
{
ret = mkfifo(argv[1], 0666);
if (ret == -1)
{
printf("mkfifo is error \n");
return -2;
}
printf("mkfifo is ok \n");
}
fd = open(argv[1], O_WRONLY);
while (1)
{
sleep(1);
write(fd, "hello", 5);
}
close(fd);
return 0;
}
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <sys/wait.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <string.h>
int main(int argc, char *argv[])
{
char buf[32] = {0};
int fd;
if (argc < 2)
{
printf("Usage:%s <fifo name> \n", argv[0]);
return -1;
}
fd = open(argv[1], O_RDONLY);
while (1)
{
sleep(1);
read(fd, buf, 32);
printf("buf is %s\n", buf);
memset(buf, 0, sizeof(buf));
}
close(fd);
return 0;
}
4.结果
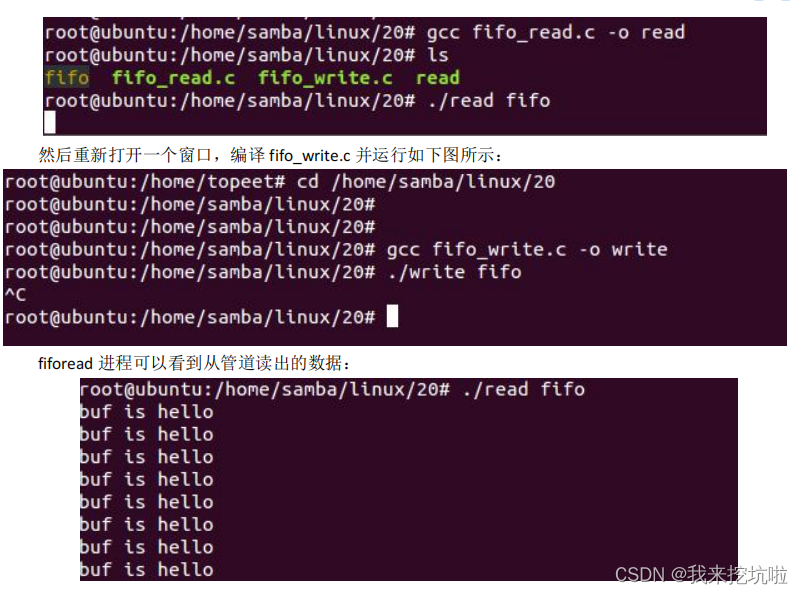
二.共享内存
共享内存是最快的通信方式。共享内存允许多个进程访问同一块物理内存空间,以实现快速的数据共享和通信。但是,共享内存需要解决诸如数据同步和互斥等问题。
三.消息队列
消息队列是一种灵活的进程间通信方式。它允许多个进程通过发送和接收消息来进行通信。消息队列可以在不同的进程间传递数据,并且可以解决进程间的同步和互斥问题。
四.信号量
信号量是一种用于控制多个进程对共享资源的访问数量的工具。它通常用于解决并发访问共享资源的问题。
五.信号
信号是一种异步的通信方式,用于通知另一个进程某个事件已经发生或者请求终止进程等操作。
六.套接字
套接字是一种更为通用的进程间通信方式。它可以通过网络在不同的计算机之间进行通信,也可以在本地进程间进行通信。套接字可以用于实现多种类型的通信需求,包括数据传输、命名管道、共享内存等。