#include <iostream>
using namespace std;
typedef int Elemtype;
#define Maxsize 5
#define ERROR 0
#define OK 1
typedef struct
{
Elemtype data[Maxsize];
int front, rear;
}SqQueue;
void InitQueue(SqQueue& Q)
{
Q.rear = Q.front = 0;
}
bool isEmpty(SqQueue Q)
{
if (Q.rear == Q.front) return OK;
else return ERROR;
}
bool EnQueue(SqQueue& Q, Elemtype x)
{
if ((Q.rear + 1) % Maxsize == Q.front)
{
cout << "堆满啦" << endl;
return ERROR;
}
Q.data[Q.rear] = x;
Q.rear = (Q.rear + 1) % Maxsize;
return OK;
}
bool DeQueue(SqQueue& Q, Elemtype &x)
{
if (Q.rear == Q.front) return ERROR;
x = Q.data[Q.front];
Q.front = (Q.front + 1) % Maxsize;
return OK;
}
int main(void)
{
SqQueue Q;
InitQueue(Q);
EnQueue(Q, 1);
EnQueue(Q, 2);
EnQueue(Q, 3);
EnQueue(Q, 4);
for (int i = 0; i < Maxsize; i++)
printf("data[%d]=%d\n", i, Q.data[i]);
int x = 0;
EnQueue(Q, 5);
DeQueue(Q, x);
EnQueue(Q, 5);
for (int i = 0; i < Maxsize; i++)
printf("data[%d]=%d\n", i, Q.data[i]);
return 0;
}
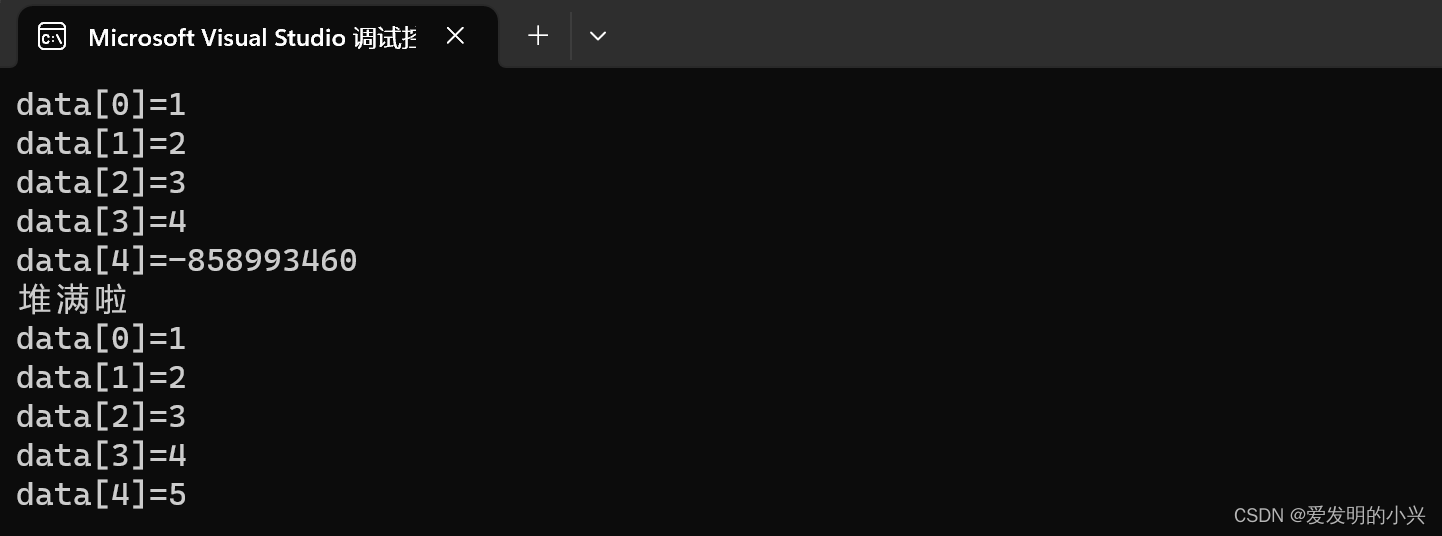