这里,App.vue中,引入了parent组件;parent组件中引入了child组件。现在要从app.vue,向child组件传值。
主要文件:
1. 通过事件总线传值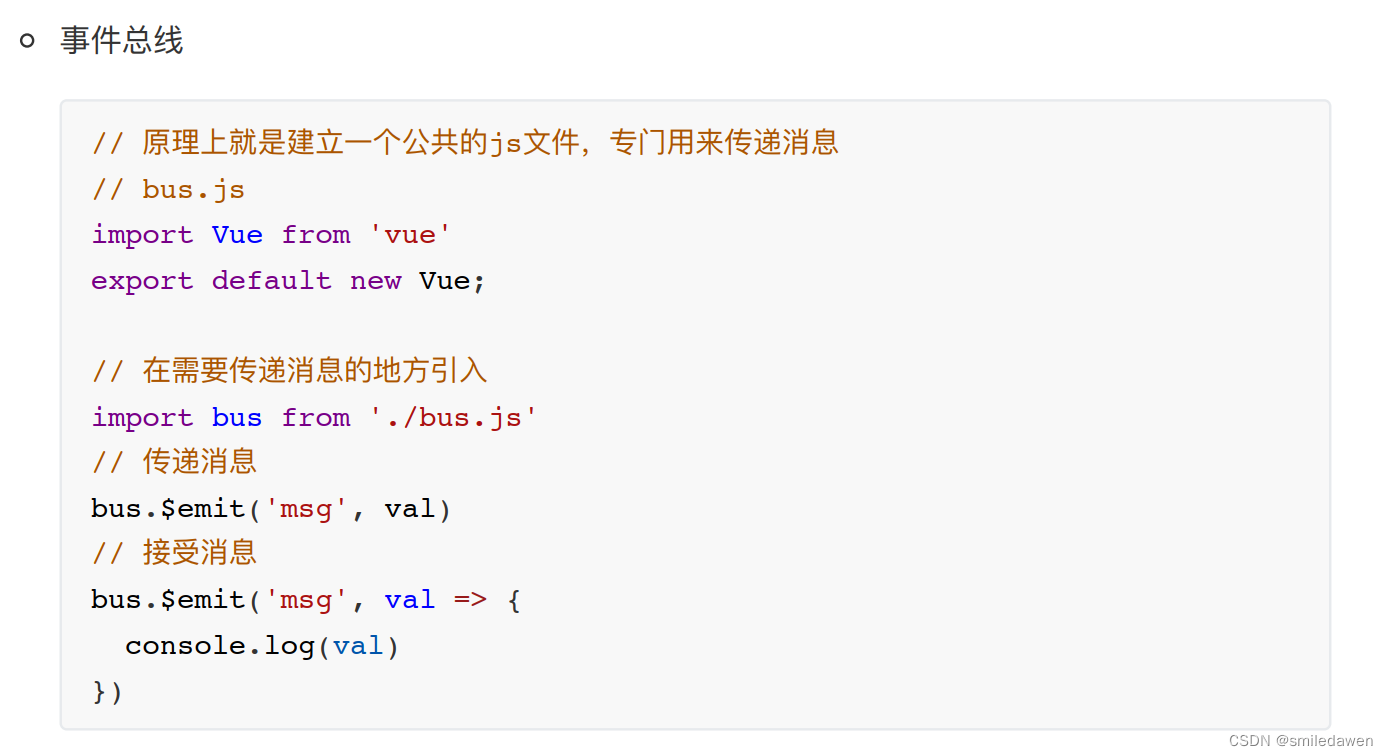
1. src ->util->bus.js
// 通过util中的bus,完成两个组件之间的数据传递
import Vue from 'vue'
export default new Vue
2. app.vue,通过bus.$emit触发事件
<!-- 非父子组件之间,通过bus进行数据传递 -->
<template>
<div id="app">
<m-parent></m-parent>
<!-- 通过button,触发事件 -->
<button @click="passMsg">go!</button>
</div>
</template>
<style>
</style>
<script>
import MParent from './views/Parent.vue'
import bus from './util/bus'
export default{
components: {
MParent,
},
methods: {
passMsg() {
// 需要往外传值的,写bus.$emit来触发事件
bus.$emit('msg','from app msg')
}
},
}
</script>
3. child.vue中,通过bus.$on接收数据
<template>
<div>
<h3>Child</h3>
<h5>{{ childMsg }}</h5>
</div>
</template>
<script>
import bus from '../util/bus'
export default {
data() {
// 新建一个变量
return {
childMsg: '',
}
},
props: {
msg: {
type: String,
default: 'child default'
},
},
mounted () {
// 监听到事件后,接收数据,保存到本地
bus.$on('msg', (val)=>{this.childMsg = val} );
},
}
</script>
<style>
</style>
4. 顺便把parent.vue内容也写过来吧
<template>
<div>
<h3>Parent</h3>
<m-child ref="child"></m-child>
</div>
</template>
<script>
import MChild from './Child.vue'
export default {
data() {
return {
msg: ''
}
},
components: {
MChild,
},
mounted () {
console.log(this.$refs.child)
},
}
</script>
<style></style>
通过attrs传值
app.vue
<template>
<div id="app">
<!-- 把本地的变量,绑定到parent组件里去 -->
<m-parent :msga="a" :msgb="b" :msgc="c"></m-parent>
</div>
</template>
<style>
</style>
<script>
import MParent from './views/Parent.vue'
import bus from './util/bus'
export default{
data() {
return {
a: 'a',
b: 'b',
c: 'c',
}
},
components: {
MParent,
},
methods: {
},
}
</script>
parent.vue
<template>
<div>
<h3>Parent</h3>
<!-- child中绑定attrs -->
<m-child v-bind="$attrs"></m-child>
</div>
</template>
<script>
import MChild from './Child.vue'
export default {
data() {
return {
msg: ''
}
},
components: {
MChild,
},
}
</script>
<style></style>
child.vue
<template>
<div>
<h3>Child</h3>
</div>
</template>
<script>
import bus from '../util/bus'
export default {
props: {
msg: {
type: String,
default: 'child default'
},
},
mounted () {
// 可以在这儿获得attrs
console.log('attrs', this.$attrs)
},
}
</script>
<style>
</style>