本文主要介绍list的一些常见接口的使用
文章目录
- 一、list的介绍
- 二、list的使用
- 2.1 list的构造函数
- 2.2 list迭代器的使用
- 2.3 list相关的容量大小相关的函数
- 2.4 list数据的访问相关的函数
- 2.5 list的数据调整相关的函数
- 2.6 list中其他函数操作
一、list的介绍
- list是可以以O(1)的时间复杂度任意位置进行插入和删除的序列式容器,并且该容器可以前后双向迭代。
- list的底层是双向循环结构,双向链表中每个元素存储在互不相关的独立节点中,在节点中通过指针指向其前一个元素和后一个元素。
- list与forward_list非常相似:最主要的不同在于forward_list是单链表,只能朝前迭代,已让其更简单高效。
- 与其他的序列式容器相比(array,vector,deque),list通常在任意位置进行插入、移除元素的执行效率更好。
- 与其他序列式容器相比,list和forward_list最大的缺陷是不支持任意位置的随机访问,比如:要访问list的第6个元素,必须从已知的位置(比如头部或者尾部)迭代到该位置,在这段位置上迭代需要线性的时间开销;list还需要一些额外的空间,以保存每个节点的相关联信息(对于存储类型较小元素的大list来说这可能是一个重要的因素)
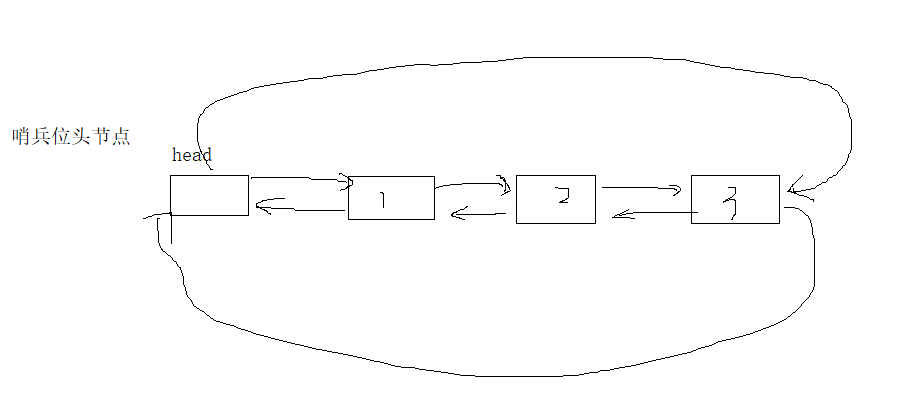
二、list的使用
2.1 list的构造函数
构造函数 ( constructor) | 接口说明 |
---|
list (size_type n, const value_type& val = value_type()) | 构造的list中包含n个值为val的元素 |
list() | 构造空的list |
list (const list& x) | 拷贝构造函数 |
list (InputIterator first, InputIterator last) | 用[first, last)区间中的元素构造list |
void TestList1()
{
list<int> l1;
list<int> l2(4, 100);
list<int> l3(l2.begin(), l2.end());
list<int> l4(l3);
int array[] = { 16,2,77,29 };
list<int> l5(array, array + sizeof(array) / sizeof(int));
list<int> l6{ 1,2,3,4,5 };
list<int>::iterator it = l5.begin();
while (it != l5.end())
{
cout << *it << " ";
++it;
}
cout << endl;
for (auto& e : l5)
cout << e << " ";
cout << endl;
}
2.2 list迭代器的使用
函数声明 | 接口说明 |
---|
begin+end | 返回第一个元素的迭代器+返回最后一个元素的下一个位置的迭代器 |
rbegin+rend | 返回end位置+返回begin位置 |
void PrintList(const list<int>& l)
{
for (list<int>::const_iterator it = l.begin(); it != l.end(); ++it)
{
cout << *it << " ";
}
cout << endl;
}
void TestList2()
{
int array[] = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 0 };
list<int> l(array, array + sizeof(array) / sizeof(array[0]));
auto it = l.begin();
while (it != l.end())
{
cout << *it << " ";
++it;
}
cout << endl;
auto rit = l.rbegin();
while (rit != l.rend())
{
cout << *rit << " ";
++rit;
}
cout << endl;
}
2.3 list相关的容量大小相关的函数
函数声明 | 接口说明 |
---|
empty | 检测list是否为空,是返回true,否返回false |
size | 返回list中有效结点的个数 |
void test_list3()
{
list<int> l1;
l1.push_back(1);
l1.push_back(2);
l1.push_back(3);
l1.push_back(4);
cout << l1.size() << endl;
cout << l1.empty() << endl;
}
2.4 list数据的访问相关的函数
函数声明 | 接口说明 |
---|
front | 返回list中的第一个结点值的引用 |
back | 返回list中最后一个结点值的引用 |
void test_list4()
{
list<int> l1;
l1.push_back(1);
l1.push_back(2);
l1.push_back(3);
l1.push_back(4);
cout << l1.front() << endl;
cout << l1.back() << endl;
}
2.5 list的数据调整相关的函数
函数声明 | 接口说明 |
---|
push_front | 在首元素前插入元素 |
pop_front | 删除第一个元素 |
push_back | 尾插 |
pop_back | 尾删 |
insert | 在pos位置插入值 |
erase | 删除pos位置的值 |
swap | 交换两个list中的值 |
clear | 清空list中的有效元素 |
注意:list迭代器支持“+”、“+=”、“<”,“++”等操作符运算,不支持[]运算符
void TestList4()
{
int array1[] = { 1, 2, 3 };
list<int> L(array1, array1 + sizeof(array1) / sizeof(array1[0]));
auto pos = ++L.begin();
cout << *pos << endl;
L.insert(pos, 4);
PrintList(L);
L.insert(pos, 5, 5);
PrintList(L);
vector<int> v{ 7, 8, 9 };
L.insert(pos, v.begin(), v.end());
PrintList(L);
L.erase(pos);
PrintList(L);
L.erase(L.begin(), L.end());
PrintList(L);
}
void TestList5()
{
int array1[] = { 1, 2, 3 };
list<int> l1(array1, array1 + sizeof(array1) / sizeof(array1[0]));
PrintList(l1);
list<int> l2;
l1.swap(l2);
PrintList(l1);
PrintList(l2);
l2.clear();
cout << l2.size() << endl;
}
2.6 list中其他函数操作
函数声明 | 接口说明 |
---|
sort | 排序 |
reverse | 逆置 |
unique | 去重(去重之前一般需要先排序) |
remove | 删除给定的一个值 |
void test_list6()
{
int arr[] = { 5,2,4,4,3,1 };
list<int> l1(arr, arr + sizeof(arr) / sizeof(arr[0]));
print_list(l1);
l1.reverse();
print_list(l1);
l1.sort();
print_list(l1);
l1.unique();
print_list(l1);
l1.remove(5);
print_list(l1);
l1.remove(6);
print_list(l1);
}