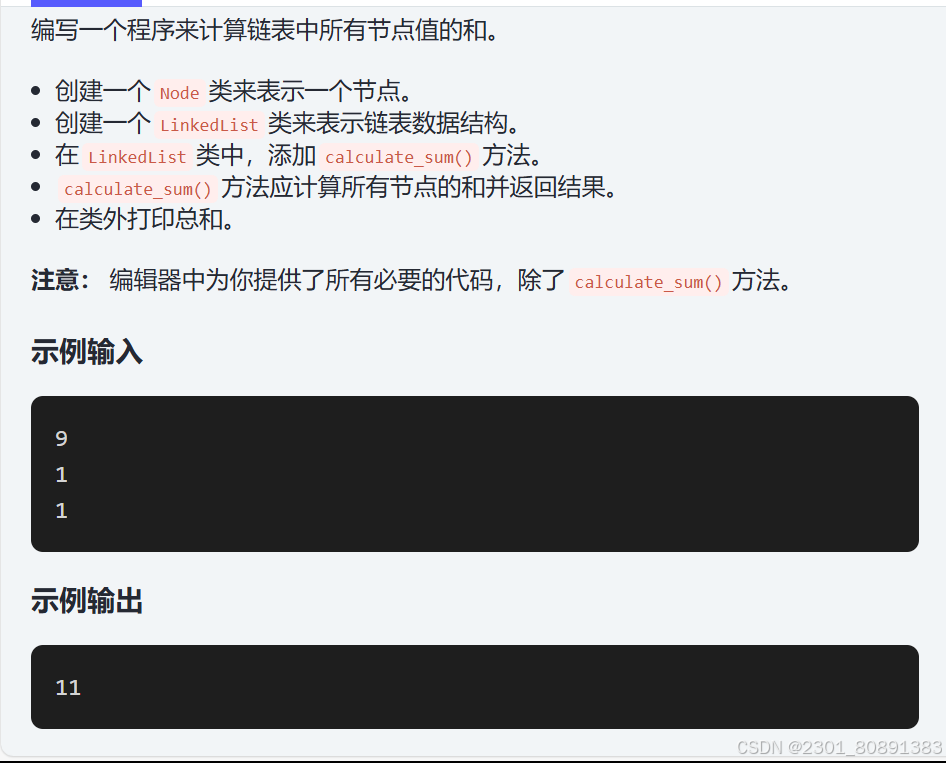
class Node:
# 节点类,每个节点包含数据(data)和指向下一个节点的引用(next)
def __init__(self, data):
self.data = data # 存储节点的数据
self.next = None # 指向下一个节点,默认值为None,表示没有下一个节点
class LinkedList:
# 链表类,包含头节点head和一些链表操作方法
def __init__(self):
self.head = None # 初始化时链表为空,head指向None
def create_linked_list(self):
# 创建链表节点并将它们连接在一起
data1 = int(input()) # 用户输入第一个节点的数据
data2 = int(input()) # 用户输入第二个节点的数据
data3 = int(input()) # 用户输入第三个节点的数据
# 创建三个节点,分别传入相应的数据
node1 = Node(data1)
node2 = Node(data2)
node3 = Node(data3)
# 将节点连接起来
self.head = node1 # 设置头节点为第一个节点
node1.next = node2 # 第一个节点指向第二个节点
node2.next = node3 # 第二个节点指向第三个节点
def calculate_sum(self):
# 计算链表中所有节点数据的和
current = self.head # 从头节点开始遍历链表
total = 0 # 初始化总和为0
# 遍历链表中的每个节点
while current:
total += current.data # 累加当前节点的数据
current = current.next # 移动到下一个节点
return total # 返回计算出的总和
# 创建一个链表实例
linked_list = LinkedList()
linked_list.create_linked_list() # 调用方法创建链表并连接节点
# 计算链表节点数据的和
total = linked_list.calculate_sum() # 调用calculate_sum方法获取总和
print(total) # 输出计算结果