2025-03-04-BeautifulSoup网络爬虫
记录BeautifulSoup网络爬虫的核心知识点
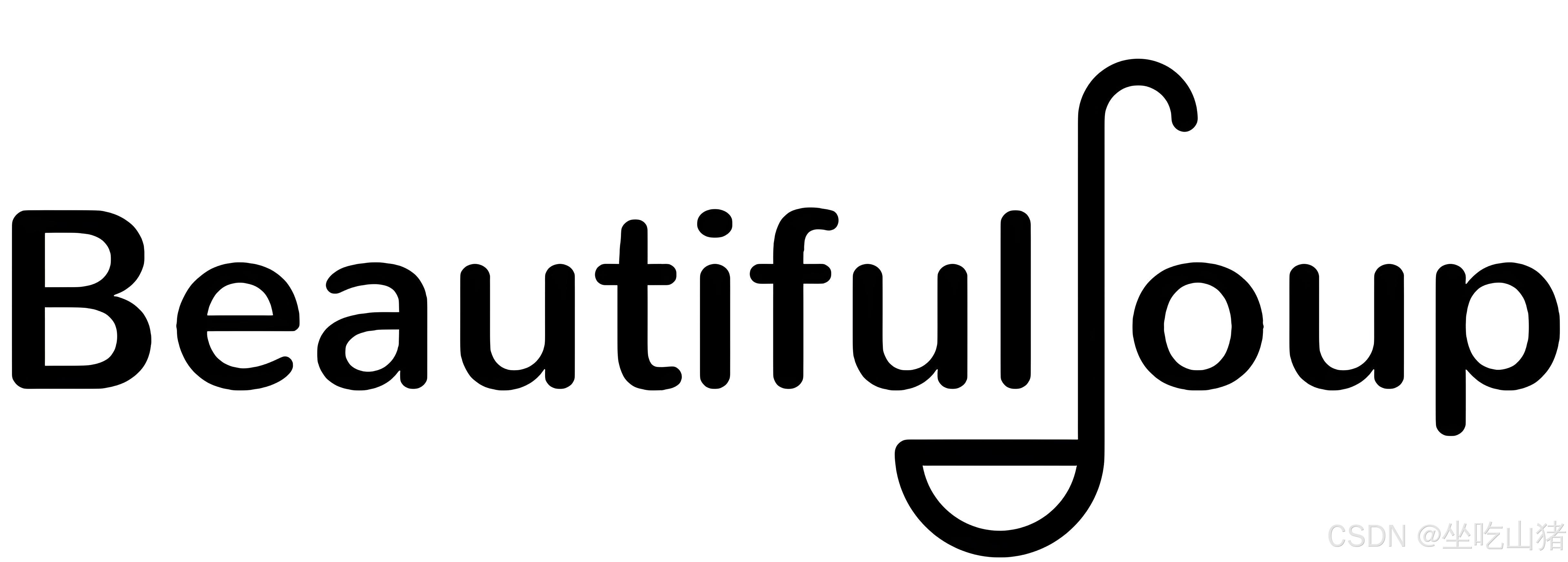
文章目录
- 2025-03-04-BeautifulSoup网络爬虫
- @[toc]
- 1-参考网址
- 2-学习要点
- 3-核心知识点
- 1. 安装
- 2. 导入必要的库
- 3. 发送 HTTP 请求
- 4. 创建 BeautifulSoup 对象
- 5. 解析 HTML 内容
- 5.1 查找标签
- 5.2 根据属性查找
- 5.3 CSS 选择器
- 6. 获取标签内容和属性
- 6.1 获取标签文本内容
- 6.2 获取标签属性值
- 7. 处理嵌套标签
- 8. 处理编码问题
- 9. 异常处理
- 10. 遵守网站规则
- 4-常用方法
- 1-属性与方法
- 2-其他属性
- 3-查找方法
- 5-代码示例
- 1-网页搜索
- 2-网页标题
- 3-网页编码
- 4-查询标签a
- 5-查询标签p
- 6-查询父标签
- 7-查询div
- 8-查询CSS
- 9-更新网页
- 10-转化网页
- 11-lxml进行XPath查找
文章目录
- 2025-03-04-BeautifulSoup网络爬虫
- @[toc]
- 1-参考网址
- 2-学习要点
- 3-核心知识点
- 1. 安装
- 2. 导入必要的库
- 3. 发送 HTTP 请求
- 4. 创建 BeautifulSoup 对象
- 5. 解析 HTML 内容
- 5.1 查找标签
- 5.2 根据属性查找
- 5.3 CSS 选择器
- 6. 获取标签内容和属性
- 6.1 获取标签文本内容
- 6.2 获取标签属性值
- 7. 处理嵌套标签
- 8. 处理编码问题
- 9. 异常处理
- 10. 遵守网站规则
- 4-常用方法
- 1-属性与方法
- 2-其他属性
- 3-查找方法
- 5-代码示例
- 1-网页搜索
- 2-网页标题
- 3-网页编码
- 4-查询标签a
- 5-查询标签p
- 6-查询父标签
- 7-查询div
- 8-查询CSS
- 9-更新网页
- 10-转化网页
- 11-lxml进行XPath查找
1-参考网址
- Python爬虫–BeautifulSoup:https://blog.csdn.net/weixin_45953332/article/details/145971342
- 个人尝试代码仓库:https://gitee.com/enzoism/beautifulsoup
2-学习要点
- 1)进行网页请求-打印网页内容
- 2)进行网页请求-确保请求成功
- 3)进行网页请求-自动检测编码
- 4)进行网页请求-find和finaAll-href属性/文本内容
- 5)进行网页请求-XPath表达式查找
- 6)进行网页请求-CSS元素捕获
- 7)进行网页请求-修改HTML内容-可修改标签的属性、文本或删除标签
- 8)进行网页请求-转换为字符串
3-核心知识点
BeautifulSoup 是一个用于从 HTML 或 XML 文件中提取数据的 Python 库,结合合适的网络请求库(如
requests
)可以方便地实现网络爬虫。以下是使用
BeautifulSoup 进行网络爬虫的核心知识点:
1. 安装
使用pip
来安装 BeautifulSoup 库,通常还需要安装解析器,常用的解析器有lxml
(速度快)和html.parser
(Python 内置)。
pip install beautifulsoup4
pip install lxml
2. 导入必要的库
在编写代码前,需要导入requests
用于发送 HTTP 请求,BeautifulSoup
用于解析 HTML 或 XML 内容。
import requests
from bs4 import BeautifulSoup
3. 发送 HTTP 请求
使用requests
库发送 HTTP 请求,获取网页的 HTML 内容。
url = 'https://example.com'
response = requests.get(url)
# 检查请求是否成功
if response.status_code == 200:
html_content = response.text
else:
print(f"请求失败,状态码: {response.status_code}")
4. 创建 BeautifulSoup 对象
使用BeautifulSoup
类创建一个解析对象,需要传入 HTML 内容和解析器名称。
soup = BeautifulSoup(html_content, 'lxml')
5. 解析 HTML 内容
5.1 查找标签
find()
:返回第一个匹配的标签对象。
# 查找第一个 <title> 标签
title_tag = soup.find('title')
print(title_tag)
find_all()
:返回所有匹配的标签对象列表。
# 查找所有 <a> 标签
all_links = soup.find_all('a')
for link in all_links:
print(link)
5.2 根据属性查找
可以通过attrs
参数或直接指定属性名来查找具有特定属性的标签。
# 查找所有 class 为 'example-class' 的 <div> 标签
divs = soup.find_all('div', class_='example-class')
for div in divs:
print(div)
# 查找所有 id 为 'example-id' 的标签
element = soup.find(id='example-id')
print(element)
5.3 CSS 选择器
使用select()
方法通过 CSS 选择器来查找标签。
# 查找所有 <p> 标签下的 <a> 标签
links_in_paragraphs = soup.select('p a')
for link in links_in_paragraphs:
print(link)
6. 获取标签内容和属性
6.1 获取标签文本内容
使用get_text()
或text
属性获取标签的文本内容。
# 获取 <title> 标签的文本内容
title_text = title_tag.get_text()
print(title_text)
6.2 获取标签属性值
使用字典索引的方式获取标签的属性值。
# 获取第一个 <a> 标签的 href 属性值
first_link = soup.find('a')
if first_link:
href_value = first_link['href']
print(href_value)
7. 处理嵌套标签
BeautifulSoup 可以方便地处理嵌套标签,通过层层查找获取所需信息。
# 查找一个包含多个 <li> 标签的 <ul> 标签
ul_tag = soup.find('ul')
if ul_tag:
li_tags = ul_tag.find_all('li')
for li in li_tags:
print(li.get_text())
8. 处理编码问题
在处理不同编码的网页时,可能需要指定编码方式。
response = requests.get(url)
response.encoding = 'utf-8' # 指定编码方式
html_content = response.text
9. 异常处理
在网络请求和解析过程中,可能会出现各种异常,需要进行适当的异常处理。
try:
response = requests.get(url)
response.raise_for_status() # 检查请求是否成功
html_content = response.text
soup = BeautifulSoup(html_content, 'lxml')
# 进行后续解析操作
except requests.RequestException as e:
print(f"请求出错: {e}")
except Exception as e:
print(f"发生其他错误: {e}")
10. 遵守网站规则
在进行网络爬虫时,需要遵守网站的robots.txt
规则,避免对网站造成过大压力。可以使用robotparser
库来检查是否可以访问某个页面。
from urllib.robotparser import RobotFileParser
rp = RobotFileParser()
rp.set_url('https://example.com/robots.txt')
rp.read()
if rp.can_fetch('*', 'https://example.com'):
# 可以进行爬取操作
pass
else:
print("不允许爬取该页面")
4-常用方法
1-属性与方法
以下是 BeautifulSoup 中常用的属性和方法:
方法/属性 | 描述 | 示例 |
---|---|---|
BeautifulSoup() | 用于解析 HTML 或 XML 文档并返回一个 BeautifulSoup 对象。 | soup = BeautifulSoup(html_doc, 'html.parser') |
.prettify() | 格式化并美化文档内容,生成结构化的字符串。 | print(soup.prettify()) |
.find() | 查找第一个匹配的标签。 | tag = soup.find('a') |
.find_all() | 查找所有匹配的标签,返回一个列表。 | tags = soup.find_all('a') |
.find_all_next() | 查找当前标签后所有符合条件的标签。 | tags = soup.find('div').find_all_next('p') |
.find_all_previous() | 查找当前标签前所有符合条件的标签。 | tags = soup.find('div').find_all_previous('p') |
.find_parent() | 返回当前标签的父标签。 | parent = tag.find_parent() |
.find_all_parents() | 查找当前标签的所有父标签。 | parents = tag.find_all_parents() |
.find_next_sibling() | 查找当前标签的下一个兄弟标签。 | next_sibling = tag.find_next_sibling() |
.find_previous_sibling() | 查找当前标签的前一个兄弟标签。 | prev_sibling = tag.find_previous_sibling() |
.parent | 获取当前标签的父标签。 | parent = tag.parent |
.next_sibling | 获取当前标签的下一个兄弟标签。 | next_sibling = tag.next_sibling |
.previous_sibling | 获取当前标签的前一个兄弟标签。 | prev_sibling = tag.previous_sibling |
.get_text() | 提取标签内的文本内容,忽略所有 HTML 标签。 | text = tag.get_text() |
.attrs | 返回标签的所有属性,以字典形式表示。 | href = tag.attrs['href'] |
.string | 获取标签内的字符串内容。 | string_content = tag.string |
.name | 返回标签的名称。 | tag_name = tag.name |
.contents | 返回标签的所有子元素,以列表形式返回。 | children = tag.contents |
.descendants | 返回标签的所有后代元素,生成器形式。 | for child in tag.descendants: print(child) |
.parent | 获取当前标签的父标签。 | parent = tag.parent |
.previous_element | 获取当前标签的前一个元素(不包括文本)。 | prev_elem = tag.previous_element |
.next_element | 获取当前标签的下一个元素(不包括文本)。 | next_elem = tag.next_element |
.decompose() | 从树中删除当前标签及其内容。 | tag.decompose() |
.unwrap() | 移除标签本身,只保留其子内容。 | tag.unwrap() |
.insert() | 向标签内插入新标签或文本。 | tag.insert(0, new_tag) |
.insert_before() | 在当前标签前插入新标签。 | tag.insert_before(new_tag) |
.insert_after() | 在当前标签后插入新标签。 | tag.insert_after(new_tag) |
.extract() | 删除标签并返回该标签。 | extracted_tag = tag.extract() |
.replace_with() | 替换当前标签及其内容。 | tag.replace_with(new_tag) |
.has_attr() | 检查标签是否有指定的属性。 | if tag.has_attr('href'): |
.get() | 获取指定属性的值。 | href = tag.get('href') |
.clear() | 清空标签的所有内容。 | tag.clear() |
.encode() | 编码标签内容为字节流。 | encoded = tag.encode() |
.is_empty_element | 检查标签是否是空元素(例如 <br> 、<img> 等)。 | if tag.is_empty_element: |
.is_ancestor_of() | 检查当前标签是否是指定标签的祖先元素。 | if tag.is_ancestor_of(another_tag): |
.is_descendant_of() | 检查当前标签是否是指定标签的后代元素。 | if tag.is_descendant_of(another_tag): |
2-其他属性
方法/属性 | 描述 | 示例 |
---|---|---|
.style | 获取标签的内联样式。 | style = tag['style'] |
.id | 获取标签的 id 属性。 | id = tag['id'] |
.class_ | 获取标签的 class 属性。 | class_name = tag['class'] |
.string | 获取标签内部的字符串内容,忽略其他标签。 | content = tag.string |
.parent | 获取标签的父元素。 | parent = tag.parent |
3-查找方法
方法/属性 | 描述 | 示例 |
---|---|---|
find_all(string) | 使用字符串查找匹配的标签。 | tag = soup.find_all('div', class_='container') |
find_all(id) | 查找指定 id 的标签。 | tag = soup.find_all(id='main') |
find_all(attrs) | 查找具有指定属性的标签。 | tag = soup.find_all(attrs={"href": "http://example.com"}) |
5-代码示例
1-网页搜索
import requests
from bs4 import BeautifulSoup
# 使用 requests 获取网页内容
url = 'https://cn.bing.com/' # 抓取bing搜索引擎的网页内容
response = requests.get(url)
# 使用 BeautifulSoup 解析网页-推荐使用 lxml 作为解析器(速度更快)
soup = BeautifulSoup(response.text, 'lxml') # 使用 lxml 解析器
# 解析网页内容 html.parser 解析器
# soup = BeautifulSoup(response.text, ‘html.parser’)
print(soup.prettify()) # 打印网页内容
2-网页标题
import requests
from bs4 import BeautifulSoup
# 使用 requests 获取网页内容
url = 'https://cn.bing.com/' # 抓取bing搜索引擎的网页内容
# 发送HTTP请求获取网页内容
response = requests.get(url)
# 中文乱码问题
response.encoding = 'utf-8'
# 确保请求成功
if response.status_code == 200:
# 使用 BeautifulSoup 解析网页-推荐使用 lxml 作为解析器(速度更快)
soup = BeautifulSoup(response.text, 'lxml') # 使用 lxml 解析器
print(soup.prettify()) # 打印网页内容
# 查找<title>标签
title_tag = soup.find('title')
# 打印标题文本
if title_tag:
print(title_tag.get_text())
else:
print("未找到<title>标签")
else:
print('请求失败')
3-网页编码
import chardet
import requests
from bs4 import BeautifulSoup
# 使用 requests 获取网页内容
url = 'https://cn.bing.com/' # 抓取bing搜索引擎的网页内容
# 发送HTTP请求获取网页内容
response = requests.get(url)
# 使用 chardet 自动检测编码
encoding = chardet.detect(response.content)['encoding']
print(encoding)
response.encoding = encoding
# 确保请求成功
if response.status_code == 200:
# 使用 BeautifulSoup 解析网页-推荐使用 lxml 作为解析器(速度更快)
soup = BeautifulSoup(response.text, 'lxml') # 使用 lxml 解析器
print(soup.prettify()) # 打印网页内容
# 查找<title>标签
title_tag = soup.find('title')
# 打印标题文本
if title_tag:
print(title_tag.get_text())
else:
print("未找到<title>标签")
else:
print('请求失败')
4-查询标签a
import requests
from bs4 import BeautifulSoup
# 指定你想要获取标题的网站
url = 'https://www.baidu.com/' # 抓取百度搜索引擎的网页内容
# 发送HTTP请求获取网页内容
response = requests.get(url)
# 中文乱码问题
response.encoding = 'utf-8'
# 使用 lxml 解析器
soup = BeautifulSoup(response.text, 'lxml')
# 查找第一个a标签
first_link = soup.find('a')
print(first_link)
print("----------------------------")
# 获取第一个 标签的 href 属性
first_link_url = first_link.get('href')
print(first_link_url)
print("----------------------------")
# 获取第一个 标签的 文本 属性
first_link_text = first_link.text.strip()
print(first_link_text)
print("----------------------------")
# 查找所有a标签
all_links = soup.find_all('a')
print(all_links)
5-查询标签p
import requests
from bs4 import BeautifulSoup
# 指定你想要获取标题的网站
url = 'https://www.baidu.com/' # 抓取百度搜索引擎的网页内容
# 发送HTTP请求获取网页内容
response = requests.get(url)
# 中文乱码问题
response.encoding = 'utf-8'
# 使用 lxml 解析器
soup = BeautifulSoup(response.text, 'lxml')
# 获取第一个 标签中的文本内容
paragraph_text = soup.find('p').get_text()
# 获取页面中所有文本内容
all_text = soup.get_text()
print(all_text)
6-查询父标签
import requests
from bs4 import BeautifulSoup
# 指定你想要获取标题的网站
url = 'https://www.baidu.com/' # 抓取百度搜索引擎的网页内容
# 发送HTTP请求获取网页内容
response = requests.get(url)
# 中文乱码问题
response.encoding = 'utf-8'
# 使用 lxml 解析器
soup = BeautifulSoup(response.text, 'lxml')
# 查找第一个a标签
first_link = soup.find('a')
print(first_link)
print("----------------------------")
# 获取第一个 标签的 href 属性
first_link_url = first_link.get('href')
print(first_link_url)
print("----------------------------")
# 获取当前标签的父标签
parent_tag = first_link.parent
print(parent_tag.get_text())
7-查询div
import requests
from bs4 import BeautifulSoup
# 指定你想要获取标题的网站
url = 'https://www.baidu.com/' # 抓取百度搜索引擎的网页内容
# 发送HTTP请求获取网页内容
response = requests.get(url)
# 中文乱码问题
response.encoding = 'utf-8'
# 使用 lxml 解析器
soup = BeautifulSoup(response.text, 'lxml')
# 查找第一个a标签
first_link = soup.find('a')
print(first_link)
print("----------------------------")
# 查找所有 class="example-class" 的 <div> 标签
divs_with_class = soup.find_all('div', class_='example-class')
print(divs_with_class)
print("----------------------------")
# 查找具有 id="su" 的 <p> 标签
unique_input = soup.find('input', id='su')
print(unique_input)
# 获取 input 输入框的值
input_value = unique_input['value']
print(input_value)
print("----------------------------")
8-查询CSS
import requests
from bs4 import BeautifulSoup
# 指定你想要获取标题的网站
url = 'https://www.baidu.com/' # 抓取百度搜索引擎的网页内容
# 发送HTTP请求获取网页内容
response = requests.get(url)
# 中文乱码问题
response.encoding = 'utf-8'
# 使用 lxml 解析器
soup = BeautifulSoup(response.text, 'lxml')
# 查找第一个a标签
first_link = soup.find('a')
print(first_link)
print("----------------------------")
# 使用CSS选择器-查找所有 class 为 'example' 的 <div> 标签
example_divs = soup.select('div.example')
print("----------------------------")
# 使用CSS选择器-查找所有 <a> 标签中的 href 属性
links = soup.select('a[href]')
print(links)
print("----------------------------")
# 使用CSS选择器-查找嵌套的 <div> 标签
nested_divs = soup.find_all('div', class_='nested')
for div in nested_divs:
print(div.get_text())
9-更新网页
import requests
from bs4 import BeautifulSoup
# 指定你想要获取标题的网站
url = 'https://www.baidu.com/' # 抓取百度搜索引擎的网页内容
# 发送HTTP请求获取网页内容
response = requests.get(url)
# 中文乱码问题
response.encoding = 'utf-8'
# 使用 lxml 解析器
soup = BeautifulSoup(response.text, 'lxml')
# 查找第一个a标签
first_link = soup.find('a')
print(first_link)
print("----------------------------")
# 获取第一个 标签的 href 属性
first_link_url = first_link.get('href')
print(first_link_url)
# 修改第一个 标签的 href 属性
first_link['href'] = 'http://popyu.com'
# 再次打印标签的 href 属性
first_link_url = first_link.get('href')
print(first_link_url)
print("----------------------------")
10-转化网页
import requests
from bs4 import BeautifulSoup
# 指定你想要获取标题的网站
url = 'https://www.baidu.com/' # 抓取百度搜索引擎的网页内容
# 发送HTTP请求获取网页内容
response = requests.get(url)
# 中文乱码问题
response.encoding = 'utf-8'
# 使用 lxml 解析器
soup = BeautifulSoup(response.text, 'lxml')
# 转换为字符串
html_str = str(soup)
print(html_str)
print("----------------------------")
11-lxml进行XPath查找
from lxml import etree
# 定义 HTML 片段
html_content = '<a class="mnav" href="http://news.baidu.com" name="tj_trnews">新闻</a>'
# 使用 lxml 的 HTML 解析器解析 HTML 内容
parser = etree.HTMLParser()
tree = etree.fromstring(html_content, parser)
# 使用 XPath 表达式查找 <a> 标签,并提取其文本内容
a_tag = tree.xpath('//a[@class="mnav"]')
if a_tag:
text = a_tag[0].text
print(text)
else:
print("未找到匹配的 <a> 标签。")