文章目录
- 一、DIP原则深度解析
- 1.1 核心定义
- 1.2 现实比喻
- 二、Spring中的DIP实现机制
- 2.1 传统实现 vs Spring实现对比
- 三、Spring中DIP的完整示例
- 3.1 领域模型定义
- 3.2 具体实现
- 3.3 高层业务类
- 3.4 配置类
- 四、Spring实现DIP的关键技术
- 4.1 依赖注入方式对比
- 4.2 自动装配注解
- 五、DIP在Spring中的实践建议
- 六、典型应用场景
- 6.1 数据库切换
- 6.2 多支付渠道
- 七、常见误区及规避
- 八、Spring Boot中的最佳实践
- 九、总结
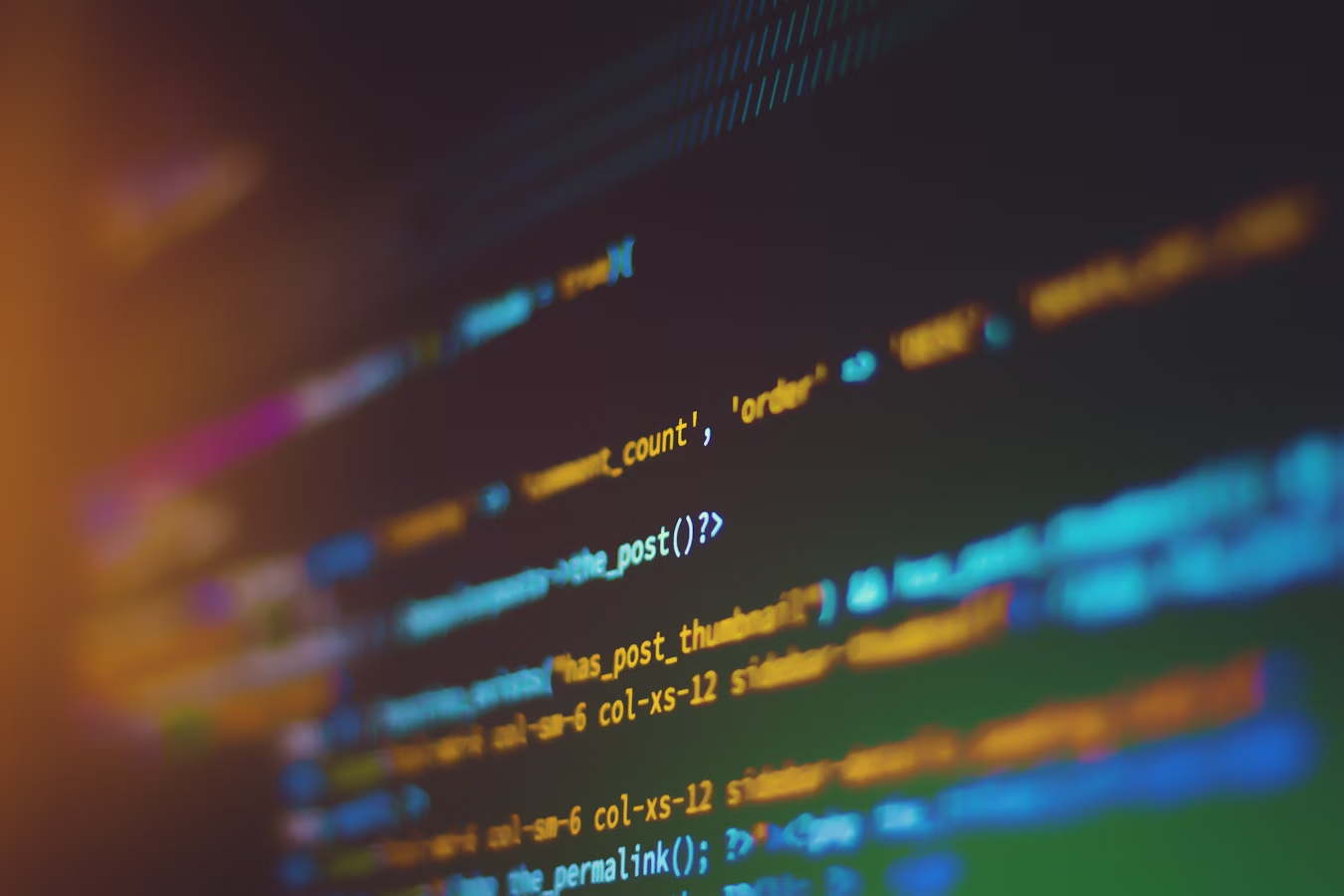
一、DIP原则深度解析
1.1 核心定义
依赖倒转原则(Dependency Inversion Principle) :高层模块不应该依赖低层模块,二者都应该依赖抽象 。抽象不应该依赖细节,细节应该依赖抽象。
1.2 现实比喻
想象一家智能家居系统:
-
高层模块:智能家居控制中心(业务逻辑)
-
低层模块:具体设备(灯泡、空调、摄像头)
-
抽象接口:统一设备控制协议
-
控制中心通过标准协议控制设备,无需关心具体设备型号。新增设备只需实现协议接口,无需修改控制中心代码。
二、Spring中的DIP实现机制
Spring框架通过两大核心功能实现DIP:
- 控制反转(IoC):将对象创建权交给容器
- 依赖注入(DI):通过构造函数/Setter/字段注入依赖
2.1 传统实现 vs Spring实现对比
// 传统方式(违反DIP)
public class OrderService {
private MySQLOrderDao orderDao = new MySQLOrderDao(); // 直接依赖具体实现
public void createOrder() {
orderDao.save();
}
}
// Spring实现(符合DIP)
@Service
public class OrderService {
private final OrderRepository orderRepository; // 依赖抽象接口
@Autowired
public OrderService(OrderRepository orderRepository) {
this.orderRepository = orderRepository;
}
}
@Repository
public class JpaOrderRepository implements OrderRepository {
// 实现接口
}
三、Spring中DIP的完整示例
3.1 领域模型定义
// 抽象层
public interface PaymentGateway {
void processPayment(BigDecimal amount);
}
public interface NotificationService {
void sendNotification(String message);
}
3.2 具体实现
// 支付实现
@Component("alipay")
public class AlipayGateway implements PaymentGateway {
@Override
public void processPayment(BigDecimal amount) {
System.out.println("支付宝支付:" + amount);
}
}
// 通知实现
@Component
public class EmailNotification implements NotificationService {
@Override
public void sendNotification(String message) {
System.out.println("发送邮件:" + message);
}
}
3.3 高层业务类
@Service
public class OrderProcessingService {
private final PaymentGateway paymentGateway;
private final NotificationService notificationService;
@Autowired
public OrderProcessingService(
@Qualifier("alipay") PaymentGateway paymentGateway,
NotificationService notificationService) {
this.paymentGateway = paymentGateway;
this.notificationService = notificationService;
}
public void completeOrder(Order order) {
paymentGateway.processPayment(order.getTotal());
notificationService.sendNotification("订单完成,金额:" + order.getTotal());
}
}
3.4 配置类
@Configuration
@ComponentScan(basePackages = "com.example")
public class AppConfig {
// 可通过@Bean配置更复杂的依赖关系
}
四、Spring实现DIP的关键技术
4.1 依赖注入方式对比
注入方式 | 代码示例 | 适用场景 |
---|---|---|
构造器注入 | @Autowired OrderService(OrderRepository repo) | 推荐首选方式,保证不可变 |
Setter注入 | @Autowired void setRepo(OrderRepository repo) | 可选依赖 |
字段注入 | @Autowired private OrderRepository repo; | 不推荐,测试困难 |
4.2 自动装配注解
@Autowired
:按类型自动装配@Qualifier
:指定具体实现bean@Primary
:设置优先注入的bean
五、DIP在Spring中的实践建议
-
接口先行 :先定义抽象接口,再实现具体类
// 先定义仓库接口 public interface UserRepository { User findById(Long id); void save(User user); } // 再实现JPA版本 @Repository public class JpaUserRepository implements UserRepository { // 具体实现 }
-
善用Profile配置 :不同环境切换实现类
@Profile("dev") @Service public class MockPaymentService implements PaymentService {} @Profile("prod") @Service public class RealPaymentService implements PaymentService {}
-
循环依赖处理:使用Setter注入或
@Lazy
注解打破循环依赖@Service public class ServiceA { private final ServiceB serviceB; @Autowired public ServiceA(@Lazy ServiceB serviceB) { this.serviceB = serviceB; } }
六、典型应用场景
6.1 数据库切换
// 通用仓库接口
public interface ProductRepository extends JpaRepository<Product, Long> {}
// MySQL实现
@Profile("mysql")
@Repository
public interface MysqlProductRepository extends ProductRepository {}
// MongoDB实现
@Profile("mongodb")
@Repository
public interface MongoProductRepository extends ProductRepository {}
6.2 多支付渠道
@Component
public class PaymentGatewayRouter {
private final Map<String, PaymentGateway> gateways;
@Autowired
public PaymentGatewayRouter(List<PaymentGateway> gatewayList) {
this.gateways = gatewayList.stream()
.collect(Collectors.toMap(
g -> g.getClass().getAnnotation(GatewayType.class).value(),
Function.identity()
));
}
public PaymentGateway getGateway(String type) {
return gateways.get(type);
}
}
七、常见误区及规避
- 过度抽象:为每个类都创建接口 -> 仅在确实需要多种实现时创建接口
- 忽略单实现:单个实现类也要通过接口注入 -> 保持架构一致性
- 滥用@Autowired:在工具类中直接注入 -> 优先使用构造函数注入
- 循环依赖:A依赖B,B又依赖A -> 使用@Lazy或重构代码结构
八、Spring Boot中的最佳实践
// 自动配置示例
@Configuration
@ConditionalOnClass(DataSource.class)
public class DatabaseAutoConfiguration {
@Bean
@ConditionalOnProperty(name = "db.type", havingValue = "mysql")
public DataSource mysqlDataSource() {
return new MysqlDataSource();
}
@Bean
@ConditionalOnProperty(name = "db.type", havingValue = "postgres")
public DataSource postgresDataSource() {
return new PostgresDataSource();
}
}
- 通过Spring Boot的条件注解,实现不同环境自动装配不同的实现类,完美体现DIP原则。
九、总结
在Spring框架中实践DIP原则的关键:
✅ 通过接口定义抽象契约
✅ 利用依赖注入解耦组件
✅ 善用Spring的自动装配机制
✅ 保持适度的抽象层级
✅ 结合设计模式增强扩展性
掌握这些技巧,Spring应用将具备:
- 更高的可测试性
- 更好的可维护性
- 更强的扩展能力
- 更清晰的架构分层
- 记住:依赖倒转不是目标,而是实现软件高质量设计的手段。在实际开发中,要平衡原则与实践需求,避免陷入过度设计的陷阱。