本节目标
1.异常概念与体系结构
2.异常的处理方式
3.异常的处理流程
4.自定义异常类
1.异常的概念与体系结构
1.1异常的概念
在Java中,将程序执行过程中发生的不正常行为称为异常,比如:
1.算术异常
System.out.println(10/0);
//执行结果
Exception in thread "main" java.lang.ArithmeticException: / by zero
2.数组越界异常
int[] arr = {1,2,3};
System.out.println(arr[100]);
//执行结果
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: 100
3.空指针异常
int[] arr = null;
System.out.println(arr.length);
//执行结果
Exception in thread "main" java.lang.NullPointerException
从上述过程中可以看到,Java中不同类型的异常,都有与其对应的类来进行描述
1.2异常的体系结构
异常种类繁多,为了对不同异常或者错误进行很好的分类管理,java内部北湖了一个异常的体系结构
从上图中可以看到:
1.Throwable:是异常体系的顶层类,其派生出两个重要的子类:Error和Exception
2.Error:指的是java虚拟机无法解决的严重问题,比如:JVM的内部错误,资源耗尽等,典型代表:
public class Person {
private String name;
private String gender;
int age;
// 想要让该类支持深拷贝,覆写Object类的clone方法即可
@Override
public Person clone() {
return (Person)super.clone();
}
}
编译时报错:
Error:(17, 35) java: 未报告的异常错误java.lang.CloneNotSupportedException; 必须对其进行捕获或声明以便抛出
2.运行时异常
boolean ret = false;
ret = 登陆游戏();
if (!ret) {
处理登陆游戏错误;
return;
}
ret = 开始匹配();
if (!ret) {
处理匹配错误;
return;
}
ret = 游戏确认();
if (!ret) {
处理游戏确认错误;
return;
}
ret = 选择英雄();
if (!ret) {
处理选择英雄错误;
return;
}
ret = 载入游戏画面();
if (!ret) {
处理载入游戏错误;
return;
}
.....
try {
登陆游戏();
开始匹配();
游戏确认();
选择英雄();
载入游戏画面();
...
} catch (登陆游戏异常) {
处理登陆游戏异常;
} catch (开始匹配异常) {
处理开始匹配异常;
} catch (游戏确认异常) {
处理游戏确认异常;
} catch (选择英雄异常) {
处理选择英雄异常;
} catch (载入游戏画面异常) {
处理载入游戏画面异常;
}
.....
throw new XXXException("异常产生的原因");
public static int getElement(int[] array,int index){
if(null==array){
throw new NullPointerException("传递的数组为null");
}
if(index<0||index>=array.length){
throw new ArrayIndexOutOfBounds Exception("传递的数组下标越界");
}
return array[index];
}
publi static void main(String[] args){
int[] array = {1,2,3};
getElement(array,3);
}
注意事项:
//语法格式
修饰符 返回值类型,方法名(参数列表)throws异常类型1,异常类型2...{
}
public class Config {
File file;
/*
FileNotFoundException : 编译时异常,表明文件不存在
此处不处理,也没有能力处理,应该将错误信息报告给调用者,让调用者检查文件名字是否给错误了
*/
public void OpenConfig(String filename) throws FileNotFoundException{
if(filename.equals("config.ini")){
throw new FileNotFoundException("配置文件名字不对");
}
// 打开文件
}
public void readConfig(){
}
}
注意事项:
1.throws必须跟在方法的参数列表之后
2.声明的异常必须是Exception后者Exception的子类
3.方法内部如果抛出多个异常,throws之后必须跟多个异常类型,之间用逗号隔开,如果抛出多个异常类型具有父子关系,则直接声明父类即可.
public class Config {
File file;
// public void OpenConfig(String filename) throws IOException,FileNotFoundException{
// FileNotFoundException 继承自 IOException
public void OpenConfig(String filename) throws IOException{
if(filename.endsWith(".ini")){
throw new IOException("文件不是.ini文件");
}
if(filename.equals("config.ini")){
throw new FileNotFoundException("配置文件名字不对");
}
// 打开文件
}
public void readConfig(){
}
}
public static void main(String[] args) throws IOException {
Config config = new Config();
config.openConfig("config.ini");
}
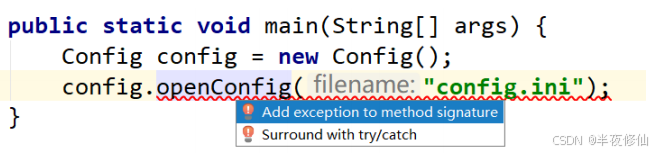
try{
//将可能出现异常的代码放在这里
}catch(要捕获的异常类型 e){
//如果try中的代码抛出异常了,此处的catch捕获异常类型与try中抛出的异常类型一致时,或者是try中抛出异常的基类时,就会被捕获到
//对异常就可以正常处理,处理完成后,跳出try-catch结构,继续执行后续代码
}[catch(异常类型 e){
//对异常进行处理
}finally{
//此处代码一定会被执行到
]}
//后续代码
//当异常被捕获到时,异常就被处理了,这里的后续代码一定会执行
//如果捕获了,由于捕获时类型不对,那就没有捕获到,这里的代码就不会被执行
注意:
1.[]中表示可选选项,可以添加,也可以不用添加
2.try中的代码可能会抛出异常,也可能不会
public class Config {
File file;
public void openConfig(String filename) throws FileNotFoundException{
if(!filename.equals("config.ini")){
throw new FileNotFoundException("配置文件名字不对");
}
// 打开文件
}
public void readConfig(){
}
public static void main(String[] args) {
Config config = new Config();
try {
config.openConfig("config.txt");
System.out.println("文件打开成功");
} catch (IOException e) {
// 异常的处理方式
//System.out.println(e.getMessage()); // 只打印异常信息
//System.out.println(e); // 打印异常类型:异常信息
e.printStackTrace(); // 打印信息最全面
}
// 一旦异常被捕获处理了,此处的代码会执行
System.out.println("异常如果被处理了,这里的代码也可以执行");
}
}
public static void main(Sting[] args){
try{
int[] array = {1,2,3};
System。out。println(array[3]);//此处会抛出数组越界异常
}catch(NullPointerException e){ //捕获时候捕获的是空指针异常,真正的异常(即抛出的数组下标越界异常)无法被捕获到
e.printStakTrace();
}
System.out.println("后序代码“); //因此后续代码并不会执行
}
Exception in thread"main"java.lang.ArrayIndexOutOfBoundsException:3
at day20210917.ArrayOperator.main(ArrayOperator.java:24)
}
※注:因为所抛出的异常(数组下标越界异常)没有相对应的catch来捕获,Java运行时环境(JRE)会认为这是一个未处理的错误情况,因此为了防止程序在错误状态下继续执行并产生不可预测的后果,JRE会终端当前线程.这是一种安全机制,确保程序不会再不稳定或错误的状态下继续运行.所以后序代码并不会执行.
3.try中可能会抛出多个不同的异常对象,则必须用多个catch来捕获----即多种异常,多次捕获
public static void main(String[] args){
int[] arr = {1,2,3};
try{
System.out.println("before");
//arr = null
System.out.println(arr[100]); //数组下标越界为空
System.out.println("after");
}catch(ArrayIndexOutOfBoundsException e){
System.ouut.println("这是个数组下标越界异常");
e.printStackTrace();
}catch(Null{ointerException e){
System.out.println("这个是空指针异常");
e.printStackTrace();
}
System.out.println("after try catch");
}
catch (ArrayIndexOutOfBoundsException | NullPointerException e) {
...
}
public static void main(String[] args){
int[] arr={1,2,3};
try{
System.out.println("before");
arr = null;
System.out.println(arr[100]);
System.out.println("after");
}catch(Exception e){ //Exception可以捕获所有的异常
e.printStackTrace();
}catch(NullPointerException){ //永远都被捕获执行到
e.printStackTrace();
}
System.out.println("after try catch");
}
Error:(33,10)java:已捕获到异常错误Java.lang.NULlPointerException
public static void main(String[] args) {
int[] arr = {1, 2, 3};
try {
System.out.println("before");
arr = null;
System.out.println(arr[100]);
System.out.println("after");
} catch (Exception e) {
e.printStackTrace();
}
System.out.println("after try catch");
}
由于Exception类是所有异常类的父类,因此可以用这个类型表示捕捉的所有异常
try{
//可能会发生异常的代码
}catch(异常类型 e){
//对捕获到的异常进行处理
}finally{
//此处的语句无论是否发生异常都会被执行到
}
//如果没有抛出异常,或者异常被捕获处理了,这里的代码也会被执行
public static void main(String[] args){
try{
int[] arr = {1,2,3};
arr[100] = 10;
arr[0] = 10;
}catch(ArrayIndexOutOfBoundsException e){
System.out.println(e);
}finally{
System.out.println("finally中的代码一定会执行");
}
System.out.println("如果没有抛出异常,或者异常被处理了,try-catch后的代码也会执行");
}
问题:既然finally和try-catch-finally后的代码都会执行,那为什么还要有finally?
需求:实现getData方法,内部输入一个整型数字,然后将该数字返回,并在main方法中打印
public class TestFinally{
public static int getData(){
Scanner sc = null;
try{
sc = new Scanner(System.in);
int data = sc.nextInt();
return data;
}catch(InputMismatchException e){
e.printStackTrace();
}finally{
System.out.println("finally中的代码");
}
System.out.println("try-catch-finally之后的代码");
if(null != sc){
sc.close();
}
return 0;
}
public static void main(String[] args){
int data = getData();
System.out.println(data);
}
}
//正常输入时程序运行结果是:
100
finally中的代码
100
上述程序,如果正常输入,成功接收输入后程序就返回了,try-catch-finally之后代码根本就没有执行,
//下面程序输出什么?
public static void main(String[] args){
System.out.println(func());
}
public static int func(){
try{
return 10;
}finally{
return 20;
}
}
A:10 B:20 C:30 D:编译失败
finally执行的时机是在方法返回之前(try或者catch中如果有return会在这个return之前执行finally中的内容).但是如果finally中也存在return语句,那么就会执行finally中的return,从而不会执行到try中原有的return.(故结果为B)
public static void main(String[] args){
try{
func();
}catch(ArrayIndexOUtOfBoundsException e){
e.printStackTrace();
}
System.out.println("after try catch");
}
public static void func(){
int[] arr = {1,2,3};
System.out.println(arr[100]);
}
//直接结果
java.lang.ArrayIndexOutOfBoundsException: 100
at demo02.Test.func(Test.java:18)
at demo02.Test.main(Test.java:9)
after try catch
如果向上一直传递都没有合适的方法处理异常,最终就会交给JVM处理,程序就会异常终止(和我们最开始未使用try-catch时是一样的).
public static void main(String[] args) {
func();
System.out.println("after try catch");
}
public static void func() {
int[] arr = {1, 2, 3};
System.out.println(arr[100]);
}
// 执行结果
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: 100
at demo02.Test.func(Test.java:14)
at demo02.Test.main(Test.java:8)
public class Logln{
private String userName = "admin";
private String password = "123456";
public static void loginInfo(String userName,String password){
if(!userName.equals(userName)){
}
if(!password.equals(password)){
}
System.out.println("登录成功");
}
public static void main(String[] args){
loginInfo("admin","123456");
}
}
class UserNameException extends Exception{
public UserNameException(String message){
super(message);
}
}
class PasswordException extends Exception{
public PasswordException(String message){
super(message);
}
}
此时我们的login代码可以改成:
public class LogIn{
private String userName = "admin";
private String password = "123456";
public static void loginInfo(String userName,String password)
throws UserNameException,PasswordException{
if(!userName.equals(uerName)){
throw new UserNameException("用户名错误");
}
if(!password.equals(password)){
throw new PasswordException("密码错误");
}
System.out.println("登录成功");
}
public static void main(String[] args){
try{
loginInfo("admin","123456");
}catch(UserNameException e){
e.printStackTrace();
}catch(PasswordException e){
e.printStackTrace();
}
}
}
注意事项:
·自定义异常通常会继承自Exception或者RuntimeException
·继承自Exception的异常默认为是受查异常
·继承自RuntimeException的异常默认是非受查异常