栈、队列都更改成模板类
栈
.hpp
#ifndef MY_STACK_H
#define MY_STACK_H
#include <iostream>
using namespace std;
template<typename T>
class my_stack
{
private:
T *base;//动态栈指针
int top;//栈顶元素
int size;//栈大小
public:
my_stack();//无参构造
my_stack(T size):top(-1),size(size)
{
base = new T[this->size];
cout<<"有参构造"<<endl;
}
~my_stack()
{
delete []base;
cout<<"析构函数"<<endl;
}
//赋值
my_stack<T> & operator=(const my_stack<T> &str);
//访问栈顶元素
T my_top();
//检查容器是否为空
bool my_empty();
//返回容纳元素数
T my_size();
//插入栈顶
void my_push(T value);
//删除栈顶
void my_pop();
};
//赋值
template<typename T>
my_stack<T> & my_stack<T>::operator=(const my_stack<T> &str)
{
if(this != &str)
{
delete []base;
this->size = str.size;
this->top = str.top;
base = new int[size];
for(int i = 0;i<size;i++)
{
base[i] = str.base[i];
}
}
return *this;
}
//访问栈顶元素
template<typename T>
T my_stack<T>::my_top()
{
if(my_empty())
{
cout<<"栈为空"<<endl;
return -1;
}
return base[top];
}
//检查容器是否为空
template<typename T>
bool my_stack<T>::my_empty()
{
return top == -1;
}
//返回容纳元素数
template<typename T>
T my_stack<T>::my_size()
{
return top+1;
}
//插入栈顶
template<typename T>
void my_stack<T>::my_push(T value)
{
if(top >= size)
{
cout<<"栈满"<<endl;
return;
}
base[++top] = value;
cout<<value<<"插入成功"<<endl;
}
//删除栈顶
template<typename T>
void my_stack<T>::my_pop()
{
if(my_empty())
{
cout<<"栈为空"<<endl;
return;
}
top--;
cout<<"删除栈顶成功"<<endl;
}
#endif // MY_STACK_H
主程序
#include "my_stack.hpp"
int main()
{
my_stack<int> s(10);
s.my_push(23);
s.my_push(45);
s.my_push(74);
cout<<"栈顶 = "<<s.my_top()<<endl;
cout<<"栈元素个数="<<s.my_size()<<endl;
s.my_pop();
cout<<"删除栈顶后栈元素个数="<<s.my_size()<<endl;
return 0;
}
队列
.hpp
#ifndef QUEUE_H
#define QUEUE_H
#include <iostream>
using namespace std;
template<typename T>
class queue
{
private:
T *base;//队列动态数组
int front;//头部
int rear;//尾部
int size;//容器大小
public:
queue();//无参构造
queue(T size):front(0),rear(0),size(size)//有参构造
{
base = new T[size];
cout<<"有参构造"<<endl;
}
//析构函数
~queue()
{
delete [] base;
cout<<"析构函数"<<endl;
}
//赋值
queue<T> &operator=(const queue<T> &str);
//访问第一个元素
T my_front();
//访问最后一个元素
T my_back();
//检查容器是否为空
bool my_empty();
//返回容器的元素数
T my_size();
//向队尾插入元素
void my_push(T value);
//删除首个插入元素
void my_pop();
};
//赋值
template<typename T>
queue<T> & queue<T>::operator=(const queue<T> &str)
{
if(this != &str)
{
delete []base;
front = str.front;
rear = str.rear;
size = str.size;
base = new int[size];
for(int i = front;i<rear;i++)
{
base[i] = str.base[i];
}
}
return *this;
}
//访问第一个元素
template<typename T>
T queue<T>::my_front()
{
if(my_empty())
{
cout<<"队列为空"<<endl;
return -1;
}
return base[front];
}
//访问最后一个元素
template<typename T>
T queue<T>::my_back()
{
if(my_empty())
{
cout<<"队列为空"<<endl;
return -1;
}
return base[rear-1];
}
//检查容器是否为空
template<typename T>
bool queue<T>::my_empty()
{
return rear == front;
}
//返回容器的元素数
template<typename T>
T queue<T>::my_size()
{
return rear-front;
}
//向队尾插入元素
template<typename T>
void queue<T>::my_push(T value)
{
base[rear++] = value;
cout<<"插入成功"<<endl;
}
//删除首个插入元素
template<typename T>
void queue<T>::my_pop()
{
if(my_empty())
{
cout<<"队列为空"<<endl;
return;
}
cout<<"首元素"<<base[front++]<<"删除成功"<<endl;
}
#endif // QUEUE_H
主程序
#include "queue.hpp"
int main()
{
queue<int> s(10);
s.my_push(14);
s.my_push(24);
s.my_push(41);
s.my_push(4);
cout<<"最后一个元素是"<<s.my_back()<<endl;
s.my_pop();
cout<<"第一个元素"<<s.my_front()<<endl;
return 0;
}
顺序表
.hpp
#ifndef SEQLIST_H
#define SEQLIST_H
#include <iostream>
#include <cstring>
using namespace std;
template<typename T>
class My_string
{
private:
T *ptr; //顺序表字符数组
int size = 15; //数组的最大
int len; //数组的实际长度
public:
My_string(); //无参构造
//有参构造
My_string(const T* src);
My_string(T num,T value);
//拷贝构造
My_string(const My_string &other);
//拷贝赋值
My_string &operator = (const My_string &other);
//析构函数
~My_string();
bool empty(); //判空
void push_back(T value); //尾插
void pop_back(); //尾删
T listsize(); //求长度
T & at(T inex); //获取任意位置元素
void clear();//清空
T *data();//返回C风格字符串
T get_length();//返回当前最大容器
void show(); //展示
void append(const T *ptr);//扩容
const My_string operator+(const My_string &other)const;//+运算符重载
T &operator[](T n);//[]运算符重载
bool operator>(const My_string &R)const;//>重载
bool operator<(const My_string &R)const;//<重载
bool operator==(const My_string &R)const;//==重载
bool operator>=(const My_string &R)const;//>=重载
bool operator<=(const My_string &R)const;//<=重载
bool operator!=(const My_string &R)const;//!=重载
My_string &operator +=(const My_string &R);//+=重载
friend ostream &operator<<(ostream &L,const My_string &R);
friend istream &operator>>(istream &L, My_string &R);
};
template<typename T>
My_string<T>::My_string():size(15) //无参构造
{
this->ptr = new char[size];
this->ptr[0] = '\0';
this->len = 0;
}
//有参构造
template<typename T>
My_string<T>::My_string(const T* src)
{
len = strlen(src)+1;
size = len >size?size*2:size;
this->ptr = new char[size];
strcpy(ptr,src);
}
template<typename T>
My_string<T>::My_string(T num,T value)
{
size = num >size?size*2:size;
ptr = new char[size];
for(int i = 0;i<num;i++)
{
this->ptr[i] = value;
}
this->len = num;
}
//拷贝构造
template<typename T>
My_string<T>::My_string(const My_string &other):ptr(new char[other.size]),size(other.size),len(other.len)
{
strcpy(this->ptr,other.ptr);
this->size = other.size;
this->len = other.len;
}
//拷贝赋值
template<typename T>
My_string<T> &My_string<T>::operator = (const My_string<T> &other)
{
if(this != &other)
{
delete []ptr;
size = other.size;
ptr = new char[size + 1];
strcpy(ptr,other.ptr);
}
return *this;
}
//析构函数
template<typename T>
My_string<T>::~My_string()
{
delete []ptr;
}
template<typename T>
T *My_string<T>::data()//返回C风格字符串
{
return ptr;
}
//判空
template<typename T>
bool My_string<T>::empty()
{
return ptr[0] == 0;
}
//尾插
template<typename T>
void My_string<T>::push_back(T value)
{
this->ptr[len++] = value;
}
//尾删
template<typename T>
void My_string<T>::pop_back()
{
if(this->empty())
{
cout<<"表为空无删除对象"<<endl;
return;
}
this->len--;
}
//求长度
template<typename T>
T My_string<T>::listsize()
{
return this->len;
}
//获取任意位置元素
template<typename T>
T & My_string<T>::at(T index)
{
if(this->empty())
{
throw std::out_of_range("表为空无对象");
}
if(index>this->len||index<=0)
{
throw std::out_of_range("位置错误");
}
return this->ptr[index-1];
}
//展示
template<typename T>
void My_string<T>::show()
{
if(this->empty())
{
cout<<"表为空无对象"<<endl;
return;
}
cout<<"当前顺序表中的元素是:";
cout<<"ptr = "<<ptr<<" ";
len = strlen(ptr);
cout<<"len = "<<len<<endl;
}
template<typename T>
T My_string<T>::get_length()//返回当前最大容器
{
return this->size;
}
template<typename T>
void My_string<T>::clear()//清空
{
delete []ptr;
ptr = new char[1];
ptr[0] = '\0';
len = 0;
}
template<typename T>
void My_string<T>::append(const T *src)
{
int src_len = strlen(src);
while(len+src_len >= size)
{
size *= 2;
char *new_ptr = new char[size];
strcpy(new_ptr,ptr);
delete []ptr;
ptr = new_ptr;
}
strcat(ptr,src);
len += src_len;
}
template<typename T>
const My_string<T> My_string<T>::operator+(const My_string<T> &other)const//+运算符重载
{
My_string temp;
delete []temp.ptr;
temp.len =this->len+other.len;
temp.ptr = new char(temp.len+1);
strcpy(temp.ptr,this->ptr);
strcat(temp.ptr,other.ptr);
return temp;
}
template<typename T>
T &My_string<T>::operator[](T n)//[]运算符重载
{
if(n<0||n>len)
{
cout<<"数组越界"<<endl;
}
else
{
return ptr[n-1];
}
}
template<typename T>
bool My_string<T>::operator>(const My_string<T> &R)const//>重载
{
return strcmp(this->ptr,R.ptr)>0;
}
template<typename T>
bool My_string<T>::operator<(const My_string<T> &R)const//<重载
{
return strcmp(this->ptr,R.ptr)<0;
}
template<typename T>
bool My_string<T>::operator==(const My_string<T> &R)const//==重载
{
return strcmp(this->ptr,R.ptr)==0;
}
template<typename T>
bool My_string<T>::operator>=(const My_string<T> &R)const//>=重载
{
return strcmp(this->ptr,R.ptr)>=0;
}
template<typename T>
bool My_string<T>::operator<=(const My_string<T> &R)const//<=重载
{
return strcmp(this->ptr,R.ptr)<=0;
}
template<typename T>
bool My_string<T>::operator!=(const My_string<T> &R)const//!=重载
{
return strcmp(this->ptr,R.ptr)!=0;
}
template<typename T>
My_string<T> &My_string<T>::operator +=(const My_string<T> &R)//+=重载
{
My_string temp;
temp.ptr = new char(len+1);
strcpy(temp.ptr,this->ptr);
temp.len = this->len;
delete [] ptr;
this->ptr = new char(temp.len+R.len+1);
strcpy(ptr,temp.ptr);
strcat(ptr,R.ptr);
return *this;
}
#endif // SEQLIST_H
主程序
#include "seqlist.hpp"
int main()
{
My_string<char> s1("Hello");
//拷贝构造
My_string<char> s2 = s1;
s2.show();
//无参构造
My_string<char> s3;
//拷贝赋值
s3 = s1;
s3.show();
s3.push_back('a');
//C字符风格
cout<<s3.data()<<endl;
s3.pop_back();
s3.append(" world ,good");
s3.show();
//求实际长度
cout<<"s3容器的实际长度 = "<<s3.listsize()<<endl;
cout<<"s3容器的最大长度 = "<<s3.get_length()<<endl;
cout<<"s3容器2号元素= "<<s3.at(2)<<endl;
//clear
s3 = s2+s1;
s3.show();
cout<<"s3[2]= "<<s3[2]<<endl;
My_string<char> s4("good");
return 0;
}
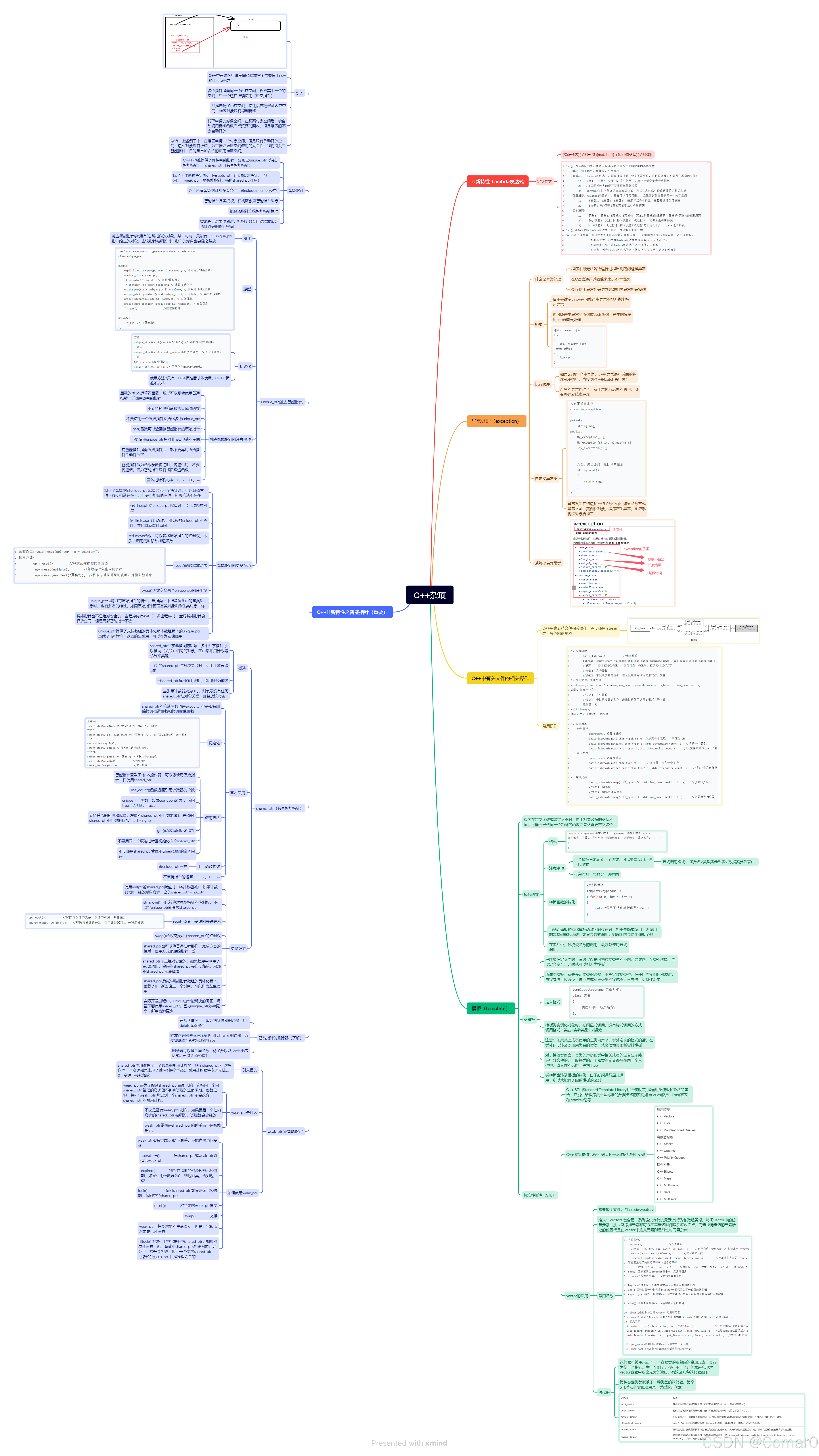