<template>
<div>
<div class="content">
<div class="but1" @click="rotateLeft">--向左</div>
<div class="ccc">
<main id="main">
<div class="haha" ref="haha">
<div
class="box"
v-for="(item, index) in imgList"
:key="index"
:style="boxStyle(index)"
>
<!-- :style="{ '--d': index }" -->
<!-- :style="boxStyle(index)" -->
<div class="boxs">{{ index }}
<img class="img" :src="item.url" alt="" />
</div>
</div>
</div>
</main>
</div>
<div class="but2" @click="rotateRight">++向右</div>
</div>
<p>当前中间的图片索引是: {{ currentIndex }}</p>
</div>
</template>
<script lang="ts" setup>
import { ref, onMounted, onUnmounted,computed } from "vue";
import img1 from "./imgs/pic_dsjfx.png";
import img2 from "./imgs/pic_lyxxw.png";
import img3 from "./imgs/pic_rjgl.png";
import img4 from "./imgs/pic_yqjc.png";
import img5 from "./imgs/pic_lyzhjgpt.png";
const haha = ref<HTMLDivElement | null>(null);
const zhuan = ref(0);
let lunbo: number | undefined;
const imgList = [
{
url: img4,
},
{
url: img5,
},
{
url: img1,
},
{
url: img2,
},
{
url: img3,
},
{
url: img4,
},
{
url: img5,
},
{
url: img1,
},
{
url: img2,
},
];
const boxes = [
{ background: "orange" },
// { background: 'pink' },
{ background: "green" },
{ background: "red" },
{ background: "blue" },
{ background: "aqua" },
];
const rotateRight = () => {
zhuan.value -= 20;
console.log(zhuan.value);
if (haha.value) {
haha.value.style.transform = `rotateY(${zhuan.value}deg)`;
}
};
const rotateLeft = () => {
zhuan.value += 20;
console.log(zhuan.value);
if (haha.value) {
haha.value.style.transform = `rotateY(${zhuan.value}deg)`;
}
};
const startInterval = () => {
lunbo = setInterval(rotateRight, 3000);
};
const clearInterval = () => {
if (lunbo !== undefined) {
window.clearInterval(lunbo);
}
};
let chushidushi = 0
let dangqian = 0
const totalBoxes = imgList.length;
// 计算当前中间的图片索引
const currentIndex = computed(() => {
// zhuan.value / 20 用于计算旋转了多少个位置
// Math.round 用于修正因浮点数运算导致的小数问题
const normalizedIndex = Math.round(zhuan.value / 20) % totalBoxes;
// 确保 index 是一个正值并且在 [0, totalBoxes-1] 范围内
console.log(-(normalizedIndex ) % totalBoxes);
dangqian = -(normalizedIndex ) % totalBoxes
return -(normalizedIndex ) % totalBoxes;
});
// 动态计算每个 box 的样式,包括大小调整
// const boxStyle = (index: number) => {
// const offset = (index - currentIndex.value + totalBoxes) % totalBoxes;
// // const offset = dangqian;
// console.log(offset,'---offset');
// let scale = 1;
// if (offset === 0) {
// scale = 1.2; // 正中间的图片放大
// } else if (offset === 1 || offset === totalBoxes - 1) {
// scale = 0.8; // 左右两侧的图片缩小
// } else {
// scale = 0.6; // 其他图片更小
// }
// return {
// '--d': index.toString(),
// transform: ` transform: rotateY(calc(var(--d) * 20deg)) translateZ(450px); scale(${scale})`,
// };
// };
const boxStyle = (index: number) => {
const offset = (index - currentIndex.value + totalBoxes) % totalBoxes;
let scale = 1;
if (offset === 0) {
scale = 1.2; // 正中间的图片放大
} else if (offset === 1 || offset === totalBoxes - 1) {
scale = 1; // 左右两侧的图片缩小
} else if(offset === 2 || offset === totalBoxes - 2) {
scale = 0.6; // 其他图片更小
} else {
scale = 0.2; // 其他图片更小
}
return {
'--d': index.toString(),
transform: `rotateY(calc(var(--d) * 20deg)) translateZ(450px) scale(${scale})`,
transition: 'transform 0.5s ease',
};
};
onMounted(() => {
// startInterval();
// zhuan.value -= 20;
});
onUnmounted(() => {
// clearInterval();
});
</script>
<style scoped lang="scss">
.content {
width: 1800px;
margin: 0px auto;
// background-color: pink;
position: relative;
padding: 50px 0;
display: flex;
align-items: center;
}
.ccc {
width: 1800px;
height: 600px;
background-color: #4fb3f6;
margin: 0 auto;
display:flex;
align-items:center;
justify-content: center
}
main {
position: relative;
width: 130px;
height: 130px;
// margin: auto;
perspective: 900px;
background-color: rgb(242, 171, 232);
}
.haha {
position: relative;
width: 100%;
height: 100%;
transform-style: preserve-3d;
transition: all 1s;
}
.but1,
.but2 {
width: 50px;
height: 50px;
background-color: greenyellow;
}
.box {
position: absolute;
width: 100%;
height: 100%;
transform: rotateY(calc(var(--d) * 20deg)) translateZ(450px);
background-color: #d0c2c2ac;
display: flex;
justify-content: center;
}
.boxs {
width: 100px;
height: 160px;
}
.img {
width: 100%;
height: 100%;
}
</style>
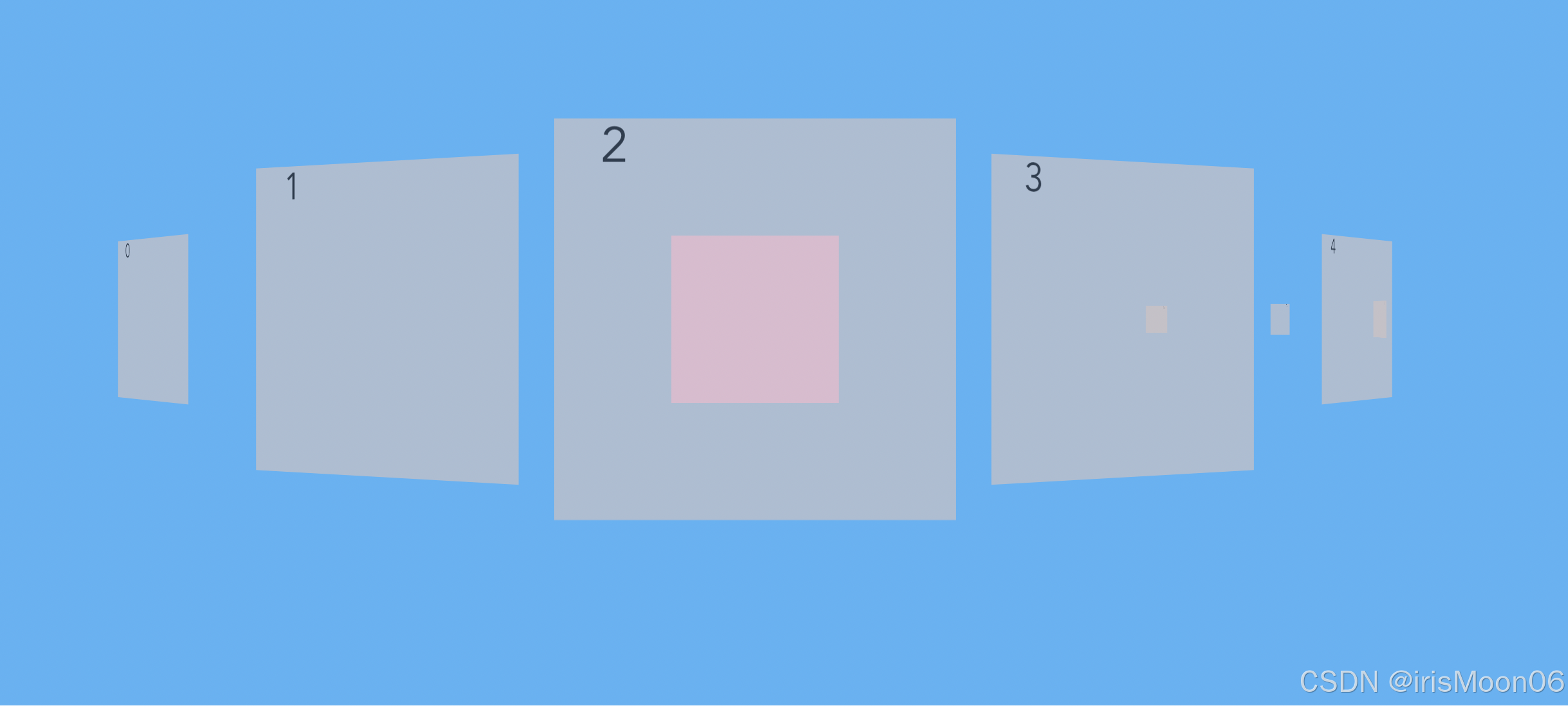