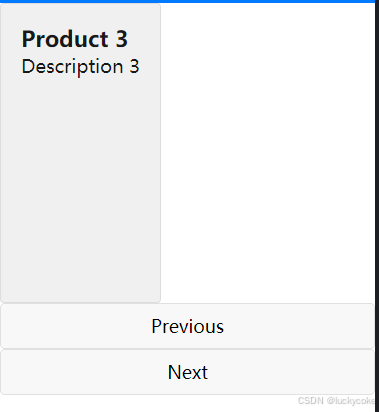
这是组件
<template>
<div class="content-wrapper">
<div
v-for="(vla, i) in products"
:key="i"
:class="['content-page', getPageClass(i)]"
>
<slot :data="vla">
<!-- 用户自定义的内容 -->
</slot>
</div>
</div>
</template>
<script>
export default {
props: {
products: {
type: Array,
required: true
},
selectedIndex: {
type: Number,
default: 0
}
},
methods: {
getPageClass(index) {
if (index === this.selectedIndex) {
return 'active';
} else if (index < this.selectedIndex) {
return 'left';
} else {
return 'right';
}
}
}
};
</script>
<style scoped>
.content-wrapper {
position: relative;
overflow: hidden;
height: 300px; /* 根据需要调整高度 */
}
.content-page {
position: absolute;
width: 100%;
height: 100%;
top: 0;
left: 0;
transition: all 0.3s ease;
opacity: 0;
transform: translateX(100%);
display: flex;
}
.content-page.active {
opacity: 1;
transform: translateX(0);
}
.content-page.left {
transform: translateX(-100%);
}
.content-page.right {
transform: translateX(100%);
}
</style>
demo 组件调用模拟
<template>
<div>
<ProductSlider :products="products" :selectedIndex="selectedIndex">
<template v-slot:default="{ data }">
<div class="product-item">
<h3>{{ data.name }}</h3>
<p>{{ data.description }}</p>
</div>
</template>
</ProductSlider>
<button @click="prev">Previous</button>
<button @click="next">Next</button>
</div>
</template>
<script>
import ProductSlider from './components/content-wrapper.vue'; // 假设组件名为 ProductSlider
export default {
components: {
ProductSlider
},
data() {
return {
products: [
{ name: 'Product 1', description: 'Description 1' },
{ name: 'Product 2', description: 'Description 2' },
{ name: 'Product 3', description: 'Description 3' }
],
selectedIndex: 0
};
},
methods: {
prev() {
if (this.selectedIndex > 0) {
this.selectedIndex--;
}
},
next() {
if (this.selectedIndex < this.products.length - 1) {
this.selectedIndex++;
}
}
}
};
</script>
<style scoped>
.product-item {
padding: 20px;
background: #f0f0f0;
border: 1px solid #ddd;
border-radius: 4px;
}
</style>