使用系统I/O实现Linux的cat命令
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <errno.h>
int main(int argc, const char *argv[])
{
if(argc < 2){
printf("请输入至少一个参数\n");
return -1;
}
int fd=0;
char buf[10]={0};
ssize_t n=0;
for(int i =1;i<argc;i++){
fd=open(argv[i],O_RDWR);
if(fd == -1){
printf("打开%s失败,错误为%s\n", argv[i], strerror(errno));
close(fd);
}
while(1){
memset(buf,0,sizeof(buf));
n=read(fd,buf,sizeof(buf)-1);
if(n == -1){
printf("文件失败 错误为%s\n", strerror(errno));
return -1;
}
printf("%s",buf);
if(n==0)break;
}
putchar(10);
}
close(fd);
return 0;
}
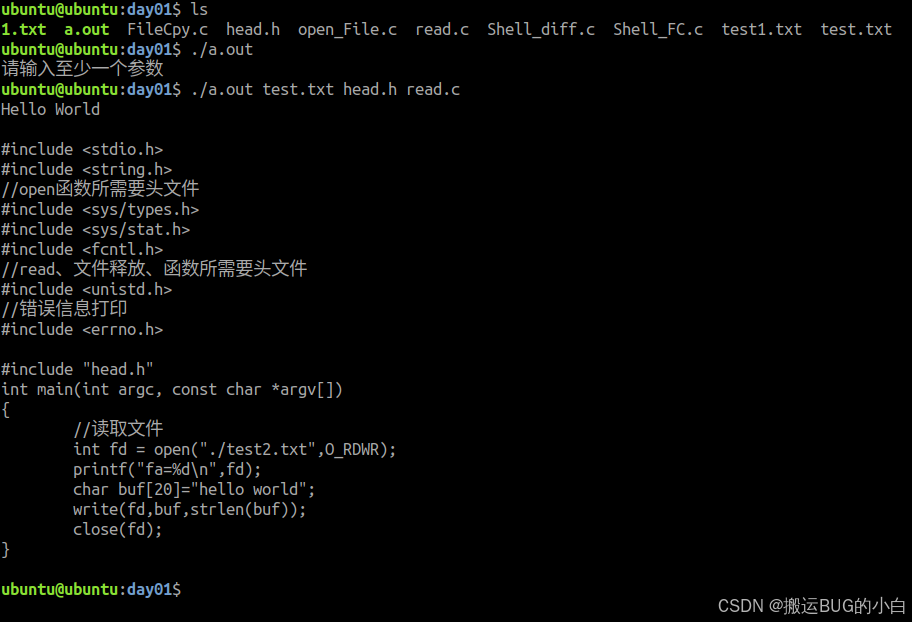
使用系统I/O实现Linux的cp命令
#include <stdio.h>
#include <string.h>
//open函数所需要头文件
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
//read、文件释放、函数所需要头文件
#include <unistd.h>
//错误信息打印
#include <errno.h>
int main(int argc, const char *argv[])
{
if(argc != 3){
printf("传参错误,需要两个参数\n");
return 0;
}
//读取文件
int fd1=open(argv[1],O_RDONLY);
if(-1 == fd1)
{
printf("打开%s失败,错误为%s\n",argv[1],strerror(errno));
}
int fd2=open(argv[2],O_RDWR | O_CREAT,0777);
if(-1 == fd2)
{
printf("打开%s失败,错误为%s\n",argv[2],strerror(errno));
}
//printf("fd1=%d\tfd2=%d\n",fd1,fd2);
//读取文件
//定义一个存放数据的空间
//初始化并清空buf
char buf[10]={0};
while(1){
memset(buf,0,sizeof(buf));
ssize_t n = read(fd1,buf,sizeof(buf)-1);
//写文件
write(fd2,buf,strlen(buf));
//printf("%s",buf);
//判断文件读取完成的的标志
//n是read函数返回的字节数
if(n==0) break;
}
//putchar(10);
//关闭打开后的文件
close(fd1);
close(fd2);
return 0;
}
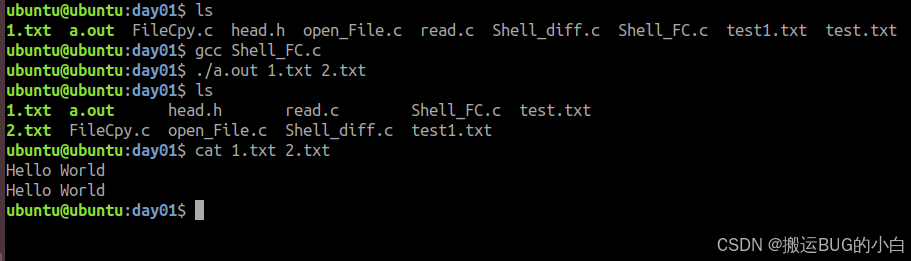
使用系统I/O实现Linux的diff命令
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <errno.h>
int main(int argc, const char *argv[])
{
if(argc != 3){
printf("传参错误,需要两个参数\n");
return 0;
}
int fd1 = open(argv[1], O_RDWR);
if(fd1 == -1){
printf("打开%s失败,错误为%s\n", argv[1], strerror(errno));
return -1;
}
int fd2 = open(argv[2], O_RDWR);
if(fd2 == -1){
printf("打开%s失败,错误为%s\n", argv[2], strerror(errno));
close(fd1);
return -1;
}
char buf[10] = {0};
char buf1[10] = {0};
while(1){
ssize_t n = read(fd1, buf, sizeof(buf) - 1);
ssize_t n1 = read(fd2, buf1, sizeof(buf1) - 1);
if(n != n1 || strcmp(buf, buf1) != 0){
printf("文件不相同\n");
break;
}
if(n == 0) break; // 文件读取完毕
}
if(n == 0 && n1 == 0){
printf("文件相同\n");
}
close(fd1);
close(fd2);
return 0;
}
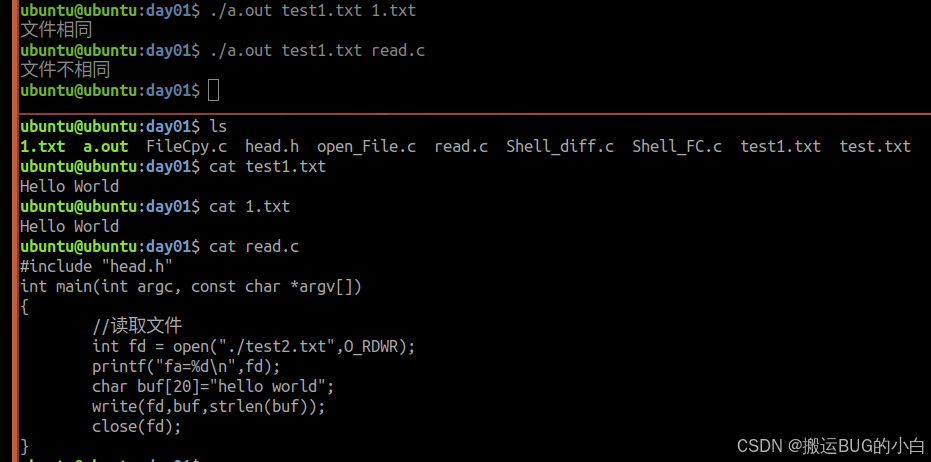