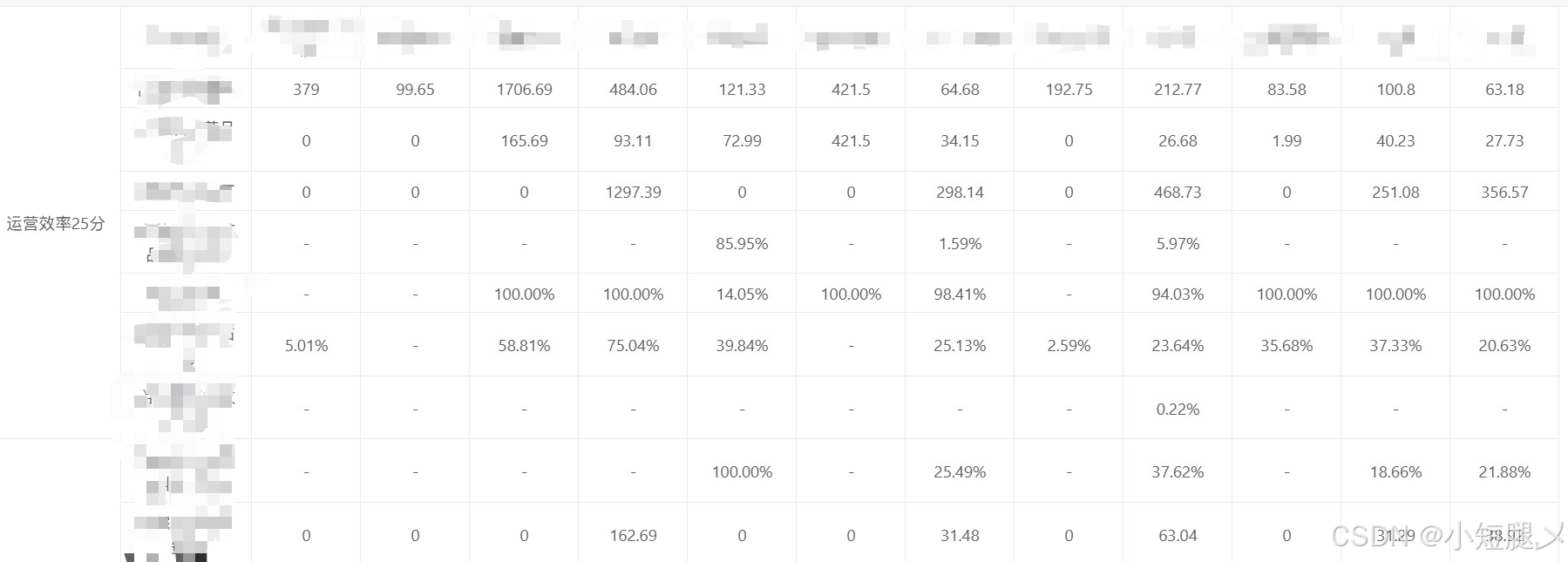
<script setup lang="ts">
import dayjs from "dayjs";
import {Search} from "@element-plus/icons-vue";
import {ElMessage} from "element-plus";
class SearchModel{
startTime?: Date | string
endTime?: Date | string
constructor() {
this.startTime = dayjs().subtract(1, 'day').startOf('day').toDate()
this.endTime = dayjs().endOf('day').toDate()
}
}
const searchModel=reactive(new SearchModel())
const isLoading=ref(false)
let myColumns = []
const result=ref(null)
async function btSearch(){
result.value=null
isLoading.value=true
myColumns=[]
const params={
}
result.value=await executeForListApi(params);
const paramsColumn={
}
const ColumnList=await DynamicApiController.selectDynamicApiColumnList(paramsColumn);
await dynamicColumn(ColumnList.data)
await reverseTable(myColumns,result.value.data)
isLoading.value=false
}
async function dynamicColumn(list){
list.map(item => {
if (item.columnName != 'deptCode')
myColumns.push( {
field : item.columnName,
title : item.columnTitle
})
})
}
async function reverseTable (columns, list) {
let index=0
const buildData = columns.map(column => {
let item = null
if(index==0 || index==8){
item = { col0 : `运营效率25分`,col1: column.title }
}else {
item = { col1: column.title }
}
index++
list.forEach((row, index) => {
item[`col${index + 2}`] = row[column.field]
})
console.log(item)
return item
})
const buildColumns = [
{
field: 'col0',
fixed: 'left',
width: 120,
align: 'center',
size: 'medium'
},
{
field: 'col1',
fixed: 'left',
width: 120,
align: 'center',
size: 'medium'
}
]
list.forEach((item, index) => {
buildColumns.push({
field: `col${index + 2}`,
fixed: '',
width: 100,
align: 'center',
size: 'medium'
})
})
gridOptions.data = buildData
gridOptions.columns = buildColumns
}
const gridOptions = reactive({
border: true,
showHeader: false,
columns: [],
data: []
})
function rowSpanMethod({row, _rowIndex, column, visibleData}) {
if (column.property == 'col0' && _rowIndex == 0) {
return {rowspan: 8, colspan: 1}
}
if (column.property == 'col0' && _rowIndex == 8) {
return {rowspan: 2, colspan: 1}
}
if (column.property == 'col0') {
return {rowspan: 0, colspan: 0}
}
return null
}
function ModifyStyle(){
let tbodies = document.getElementsByTagName('tbody');
console.log(tbodies[0])
tbodies[0].rows[0].cells[1].style.background = "red"
tbodies[0].rows[8].cells[1].style.background = "red"
}
const xTable=ref(null)
function exportDataEvent () {
if (result.value === null){
ElMessage.warning('暂无数据可打印')
return
}
xTable.value.exportData({ type: 'csv' })
}
</script>
<template>
<div>
<el-header style="padding: 0 ;margin-bottom: -10px">
<el-row :gutter="20" class="row-align">
<el-col :span="22" class="align-item">
选择日期:
<el-date-picker
type="date"
placeholder="选择开始时间"
clearable
v-model="searchModel.startTime"
/>
<span style="margin: 0 8px;">
至:
</span>
<el-date-picker
type="date"
placeholder="选择结束时间"
clearable
v-model="searchModel.endTime"
/>
</el-col>
<el-col :span="2" class="align-right">
<el-button :icon="Search" type="primary" @click="exportDataEvent">导出</el-button>
<el-button :icon="Search" type="primary" @click="btSearch">查询</el-button>
</el-col>
</el-row>
</el-header>
<el-main style="padding: 0">
<vxe-grid ref="xTable" style="font-size: 1px ! important" class="reverse-table" v-bind="gridOptions" :span-method="rowSpanMethod" height="100%" :loading="isLoading"></vxe-grid>
</el-main>
</div>
</template>
<style scoped lang="scss">
.row-align {
display: flex;
justify-content: space-between;
align-items: center;
}
.align-item{
display: flex;
align-items: center;
}
.align-right {
display: flex;
justify-content: flex-end;
}
::v-deep(.reverse-table .vxe-body--row .vxe-body--column:first-child ){
//background-color: #f8f8f9;
}
::v-deep(.vxe-table--render-default .vxe-table--body-wrapper, .vxe-table--render-default .vxe-table--footer-wrapper, .vxe-table--render-default .vxe-table--header-wrapper){
font-size: 15px;
}
</style>