探索设计模式:组合模式
- 🧐1. 概念
- 🎯2. 作用
- 📦3. 用法
- 📦3.1 绘图示例
- 📦3.2 文件示例
- 💻4. 使用场景
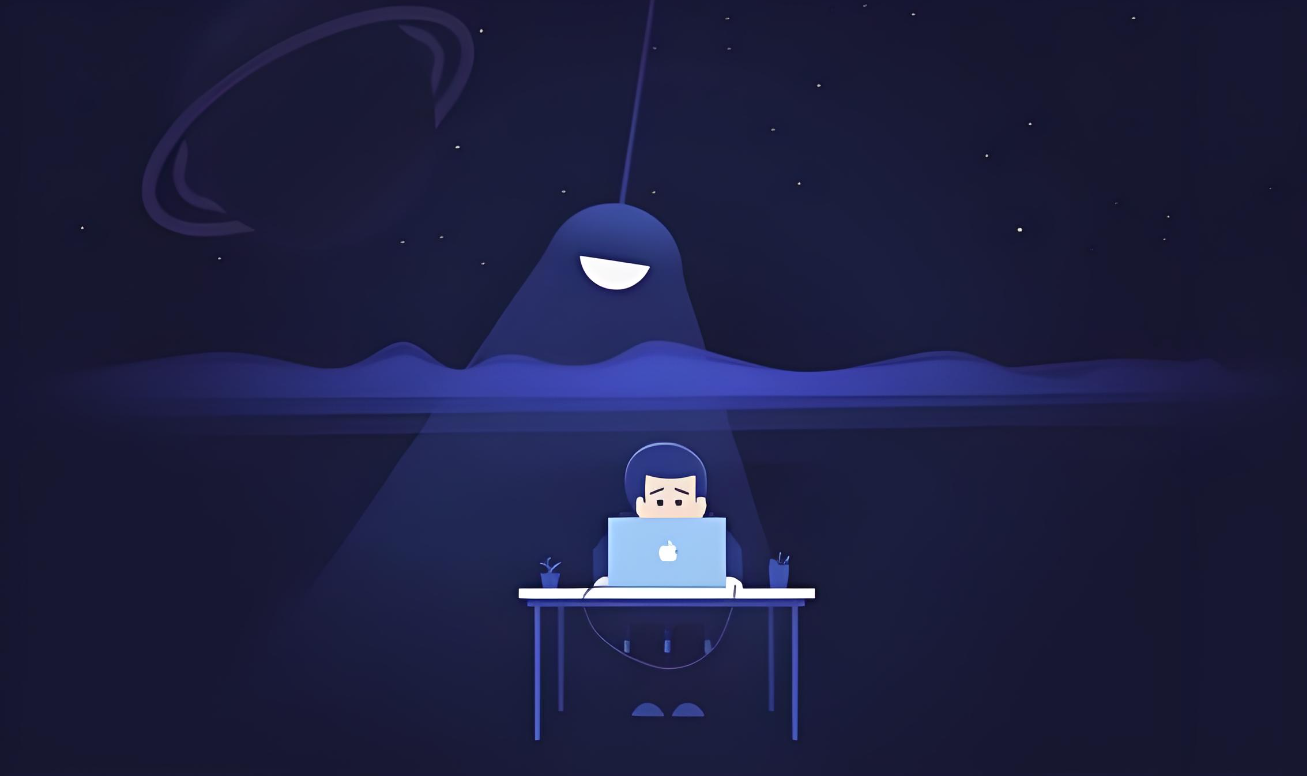
在软件设计中,组合模式(Composite Pattern
)是一种结构型设计模式,它允许你将对象组合成树形结构来表示“部分-整体
”的层次结构。这种设计模式使得客户端可以以一致的方式处理单个对象和组合对象,极大地提升了代码的灵活性和可维护性。
🧐1. 概念
组合模式由如下三个主要角色组成:
- Component(组件):这是一个接口或抽象类,定义了所有对象(包括叶子和容器)的通用行为。
- Leaf(叶子):代表树的叶节点,叶节点没有子节点。它实现了
Component
接口。 - Composite(容器):这是一个包含子节点的节点,子节点可以是叶子节点或其他容器节点。它也实现了
Component
接口,并允许对子节点进行操作,如添加、删除等。
🎯2. 作用
组合模式的主要作用:
- 统一接口:简单对象和复合对象都实现相同的接口,客户端无需关心它处理的是单个对象还是一个组合对象。
- 降低复杂性:通过树形结构的管理和操作,简化了对复杂对象图(
Object graph
)的操作。, - 增强灵活性:可以在运行时方便地增删组合内部的成员。
📦3. 用法
📦3.1 绘图示例
我们通过一个实际的代码示例来展示组合模式的用法。假设我们在开发一个绘图的应用程序,可以绘制不同类型的图形(如线条、圆形),并且可以将图形组合成复杂图形。
// Component: 定义了图形的公共操作
interface Graphic {
void draw();
}
// Leaf: 具体的图形实现(如线条)
class Line implements Graphic {
@Override
public void draw() {
System.out.println("Drawing a line");
}
}
// Leaf: 具体的图形实现(如圆形)
class Circle implements Graphic {
@Override
public void draw() {
System.out.println("Drawing a circle");
}
}
// Composite: 可以包含多个图形对象
class CompositeGraphic implements Graphic {
private final List<Graphic> childGraphics = new ArrayList<>();
public void add(Graphic graphic) {
childGraphics.add(graphic);
}
public void remove(Graphic graphic) {
childGraphics.remove(graphic);
}
@Override
public void draw() {
for (Graphic graphic : childGraphics) {
graphic.draw();
}
}
}
// 客户端代码
public class Client {
public static void main(String[] args) {
// 创建叶子节点
Graphic line1 = new Line();
Graphic circle1 = new Circle();
// 创建组合对象
CompositeGraphic compositeGraphic = new CompositeGraphic();
compositeGraphic.add(line1);
compositeGraphic.add(circle1);
// 绘制所有图形
compositeGraphic.draw();
}
}
在这个示例中,CompositeGraphic
类可以包含多个 Graphic
对象(即具体的图形如线条和圆形),并且可以像单个 Graphic
对象一样执行 draw
操作。这大大简化了客户端代码,使得客户端可以专注于“绘制”动作,而无需关心底层的实现细节。
📦3.2 文件示例
以下是具体的代码实现,展示了一个简单的文件系统结构,其中包含文件和目录。
// 组件接口:FileSystemComponent
public interface FileSystemComponent {
void showDetails(); // 显示组件的详细信息
String getName(); // 获取组件名称
int getSize(); // 获取组件大小
}
// 叶子对象:File
public class File implements FileSystemComponent {
private String name;
private int size; // 文件大小,以KB为单位
public File(String name, int size) {
this.name = name;
this.size = size;
}
@Override
public void showDetails() {
System.out.println("File: " + name + " (Size: " + size + "KB)");
}
@Override
public String getName() {
return name;
}
@Override
public int getSize() {
return size;
}
}
// 组合对象:Directory
public class Directory implements FileSystemComponent {
private String name;
private List<FileSystemComponent> components = new ArrayList<>();
public Directory(String name) {
this.name = name;
}
public void addComponent(FileSystemComponent component) {
components.add(component);
}
public void removeComponent(FileSystemComponent component) {
components.remove(component);
}
@Override
public void showDetails() {
System.out.println("Directory: " + name);
for (FileSystemComponent component : components) {
component.showDetails();
}
}
@Override
public String getName() {
return name;
}
@Override
public int getSize() {
int totalSize = 0;
for (FileSystemComponent component : components) {
totalSize += component.getSize();
}
return totalSize;
}
public FileSystemComponent findComponentByName(String componentName) {
if (name.equalsIgnoreCase(componentName)) {
return this;
}
for (FileSystemComponent component : components) {
if (component.getName().equalsIgnoreCase(componentName)) {
return component;
}
if (component instanceof Directory) {
FileSystemComponent found = ((Directory) component).findComponentByName(componentName);
if (found != null) {
return found;
}
}
}
return null; // 找不到指定名称的组件
}
}
// 客户端代码
public class Main {
public static void main(String[] args) {
// 创建文件
FileSystemComponent file1 = new File("file1.txt", 10);
FileSystemComponent file2 = new File("file2.txt", 20);
FileSystemComponent file3 = new File("file3.jpg", 50);
FileSystemComponent file4 = new File("file4.mp4", 200);
// 创建目录
Directory directory1 = new Directory("Folder1");
Directory directory2 = new Directory("Folder2");
// 组合对象添加子组件
directory1.addComponent(file1);
directory1.addComponent(file2);
directory2.addComponent(file3);
directory2.addComponent(file4);
directory2.addComponent(directory1); // 嵌套目录
// 显示详细信息
directory2.showDetails(); // 输出整个目录树的结构和内容
System.out.println("Total size of " + directory2.getName() + ": " + directory2.getSize() + "KB");
// 查找组件
FileSystemComponent found = directory2.findComponentByName("file3.jpg");
if (found != null) {
System.out.println("Found component: " + found.getName() + " (Size: " + found.getSize() + "KB)");
} else {
System.out.println("Component not found");
}
}
}
💻4. 使用场景
- 图形界面库:在图形界面开发中,UI 元素(按钮、文本框等)和容器元素(面板、窗口等)可以使用组合模式来构建复杂的用户界面。这样,可以统一处理单个元素和组合元素,使得客户端代码更简洁。
- 文件系统和目录结构:文件系统是一个经典的组合模式应用场景。文件夹可以包含文件和其他文件夹,形成一个树形结构。通过组合模式,可以一致地处理文件和文件夹,而不必在客户端代码中区分它们。
- 组织架构和人员管理:在组织架构中,部门可以包含员工和其他部门,形成一个层次结构。通过组合模式,可以一致地管理单个员工和组合部门,简化组织管理的代码。
- 菜单和菜单项:菜单系统通常包含菜单项和子菜单,可以使用组合模式来构建菜单层次结构。这样,可以一致地处理单个菜单项和包含子菜单的菜单。
一般来说,以下几个情况下可以考虑用组合模式
- 当客户端需要统一处理单个对象和组合对象时,可以使用组合模式。
- 当希望客户端忽略组合对象与单个对象的差异,统一使用相同的接口时,可以使用组合模式。
- 当希望在不同层次结构中使用相同的处理方式,并且希望动态添加或删除对象时,可以使用组合模式。