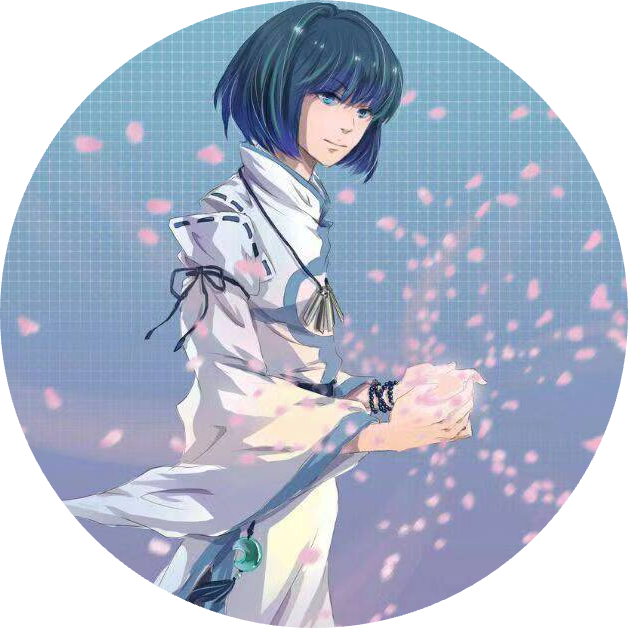

🌈个人主页: 鑫宝Code
🔥热门专栏: 闲话杂谈| 炫酷HTML | JavaScript基础
💫个人格言: "如无必要,勿增实体"
文章目录
- TypeScript函数类型:提升函数的类型安全性和可读性
- 1. 引言
- 2. 基本函数类型
- 2.1 函数声明
- 2.2 函数表达式
- 2.3 箭头函数
- 3. 可选参数和默认参数
- 3.1 可选参数
- 3.2 默认参数
- 4. 剩余参数
- 5. 函数重载
- 6. 泛型函数
- 6.1 泛型约束
- 7. 函数类型接口
- 8. 回调函数类型
- 9. 构造函数类型
- 10. 函数类型推断
- 11. 高级类型与函数
- 11.1 联合类型
- 11.2 交叉类型
- 12. 条件类型与函数
- 13. 函数类型的最佳实践
- 14. 常见陷阱和解决方案
- 14.1 this的类型
- 14.2 回调函数中的this
- 15. 实际应用示例
- 16. 结论
TypeScript函数类型:提升函数的类型安全性和可读性
1. 引言
在JavaScript中,函数是一等公民,它们可以被赋值给变量、作为参数传递,甚至作为其他函数的返回值。TypeScript作为JavaScript的超集,不仅继承了这些特性,还为函数添加了强大的类型系统支持。本文将深入探讨TypeScript中的函数类型,包括其定义、使用方法以及高级特性,帮助您更好地在TypeScript项目中使用函数,提高代码的类型安全性和可读性。
2. 基本函数类型
2.1 函数声明
在TypeScript中,我们可以为函数的参数和返回值添加类型注解:
function add(x: number, y: number): number {
return x + y;
}
2.2 函数表达式
函数表达式也可以添加类型注解:
const multiply: (x: number, y: number) => number = function(x, y) {
return x * y;
};
2.3 箭头函数
箭头函数同样支持类型注解:
const divide = (x: number, y: number): number => x / y;
3. 可选参数和默认参数
3.1 可选参数
使用?
标记可选参数:
function greet(name: string, greeting?: string): string {
return greeting ? `${greeting}, ${name}!` : `Hello, ${name}!`;
}
3.2 默认参数
默认参数可以与类型注解结合使用:
function createUser(name: string, age: number = 18): { name: string, age: number } {
return { name, age };
}
4. 剩余参数
TypeScript支持剩余参数,并可以为其指定类型:
function sum(...numbers: number[]): number {
return numbers.reduce((acc, curr) => acc + curr, 0);
}
5. 函数重载
TypeScript允许我们为同一个函数提供多个函数类型定义:
function reverse(x: string): string;
function reverse(x: number[]): number[];
function reverse(x: string | number[]): string | number[] {
if (typeof x === 'string') {
return x.split('').reverse().join('');
} else {
return x.slice().reverse();
}
}
6. 泛型函数
泛型允许我们在定义函数时不指定具体的类型,而在使用时再指定:
function identity<T>(arg: T): T {
return arg;
}
let output = identity<string>("myString");
6.1 泛型约束
我们可以使用extends关键字来约束泛型类型:
interface Lengthwise {
length: number;
}
function loggingIdentity<T extends Lengthwise>(arg: T): T {
console.log(arg.length); // Now we know it has a .length property
return arg;
}
7. 函数类型接口
我们可以使用接口来描述函数类型:
interface MathFunc {
(x: number, y: number): number;
}
let add: MathFunc = (x, y) => x + y;
let subtract: MathFunc = (x, y) => x - y;
8. 回调函数类型
在处理回调函数时,正确定义其类型非常重要:
function fetchData(callback: (data: string) => void): void {
// 模拟异步操作
setTimeout(() => {
callback("Data fetched");
}, 1000);
}
9. 构造函数类型
TypeScript允许我们定义构造函数的类型:
interface Point {
x: number;
y: number;
}
interface PointConstructor {
new (x: number, y: number): Point;
}
function createPoint(ctor: PointConstructor, x: number, y: number): Point {
return new ctor(x, y);
}
10. 函数类型推断
TypeScript能够根据上下文自动推断函数的类型:
let numbers = [1, 2, 3, 4, 5];
numbers.forEach((num) => {
console.log(num.toFixed(2)); // TypeScript knows 'num' is a number
});
11. 高级类型与函数
11.1 联合类型
函数可以接受或返回联合类型:
function formatValue(value: string | number): string {
if (typeof value === "string") {
return value.toUpperCase();
}
return value.toFixed(2);
}
11.2 交叉类型
交叉类型可以用来组合多个函数类型:
type Adder = (a: number, b: number) => number;
type Multiplier = (a: number, b: number) => number;
type Calculator = Adder & Multiplier;
const calc: Calculator = (a, b) => a + b; // 或 a * b
12. 条件类型与函数
条件类型可以根据条件选择不同的函数类型:
type FunctionType<T> = T extends string ? (arg: string) => string : (arg: number) => number;
function processValue<T extends string | number>(value: T): FunctionType<T> {
if (typeof value === "string") {
return ((arg: string) => arg.toUpperCase()) as FunctionType<T>;
} else {
return ((arg: number) => arg * 2) as FunctionType<T>;
}
}
13. 函数类型的最佳实践
-
明确指定返回类型:虽然TypeScript可以推断返回类型,但明确指定可以提高代码可读性和可维护性。
-
使用函数类型别名:对于复杂的函数类型,使用类型别名可以提高可读性:
type AsyncCallback<T> = (error: Error | null, result: T) => void;
-
利用泛型:当函数可以处理多种类型时,优先考虑使用泛型而不是any。
-
使用函数重载:当函数根据不同的参数有不同的行为时,使用函数重载可以提供更精确的类型信息。
-
避免过度使用可选参数:过多的可选参数可能导致函数调用变得复杂,考虑使用对象参数模式。
14. 常见陷阱和解决方案
14.1 this的类型
在TypeScript中,可以明确指定this的类型:
interface User {
name: string;
greet(this: User): void;
}
const user: User = {
name: "Alice",
greet() {
console.log(`Hello, I'm ${this.name}`);
}
};
14.2 回调函数中的this
在回调函数中,this的类型可能会丢失。使用箭头函数或显式绑定可以解决这个问题:
class Handler {
info: string;
onEvent(this: Handler, e: Event) {
// this的类型是Handler
this.info = e.type;
}
}
15. 实际应用示例
让我们通过一个实际的应用示例来展示TypeScript函数类型的强大功能:
// 定义一个表示HTTP方法的类型
type HttpMethod = 'GET' | 'POST' | 'PUT' | 'DELETE';
// 定义一个表示API端点的接口
interface ApiEndpoint<T> {
url: string;
method: HttpMethod;
params?: object;
response: T;
}
// 定义一个通用的API调用函数
async function apiCall<T>(endpoint: ApiEndpoint<T>): Promise<T> {
const { url, method, params } = endpoint;
const response = await fetch(url, {
method,
body: params ? JSON.stringify(params) : undefined,
headers: {
'Content-Type': 'application/json'
}
});
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
return await response.json() as T;
}
// 定义一些具体的API端点
interface User {
id: number;
name: string;
email: string;
}
const getUserEndpoint: ApiEndpoint<User> = {
url: '/api/user/1',
method: 'GET',
response: {} as User
};
const createUserEndpoint: ApiEndpoint<User> = {
url: '/api/user',
method: 'POST',
params: { name: 'New User', email: 'newuser@example.com' },
response: {} as User
};
// 使用apiCall函数
async function fetchUser() {
try {
const user = await apiCall(getUserEndpoint);
console.log('Fetched user:', user.name);
} catch (error) {
console.error('Error fetching user:', error);
}
}
async function createUser() {
try {
const newUser = await apiCall(createUserEndpoint);
console.log('Created user:', newUser.id);
} catch (error) {
console.error('Error creating user:', error);
}
}
fetchUser();
createUser();
这个例子展示了如何使用TypeScript的函数类型和泛型来创建一个类型安全的API调用系统:
- 使用类型别名和接口定义API相关的类型
- 创建一个泛型函数
apiCall
来处理不同类型的API请求 - 使用函数重载和条件类型可以进一步改进这个系统,以处理更复杂的场景
16. 结论
TypeScript的函数类型系统为JavaScript的函数添加了强大的类型检查和安全性。通过本文的介绍,我们探讨了从基本的函数类型定义到高级特性如泛型、条件类型等多个方面。掌握这些概念和技巧,将帮助您更有效地使用TypeScript,编写出更加健壮、可维护的代码。
在实际开发中,合理运用函数类型可以大大减少运行时错误,提高代码质量。随着您在项目中不断实践,您会发现TypeScript的函数类型系统不仅能捕获潜在的错误,还能提供更好的代码提示和自动完成功能,从而提高开发效率。
继续探索和实践,相信您会在TypeScript的类型系统中发现更多精彩,让您的函数更加安全、清晰和高效!
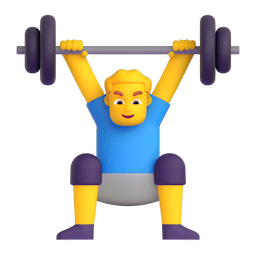