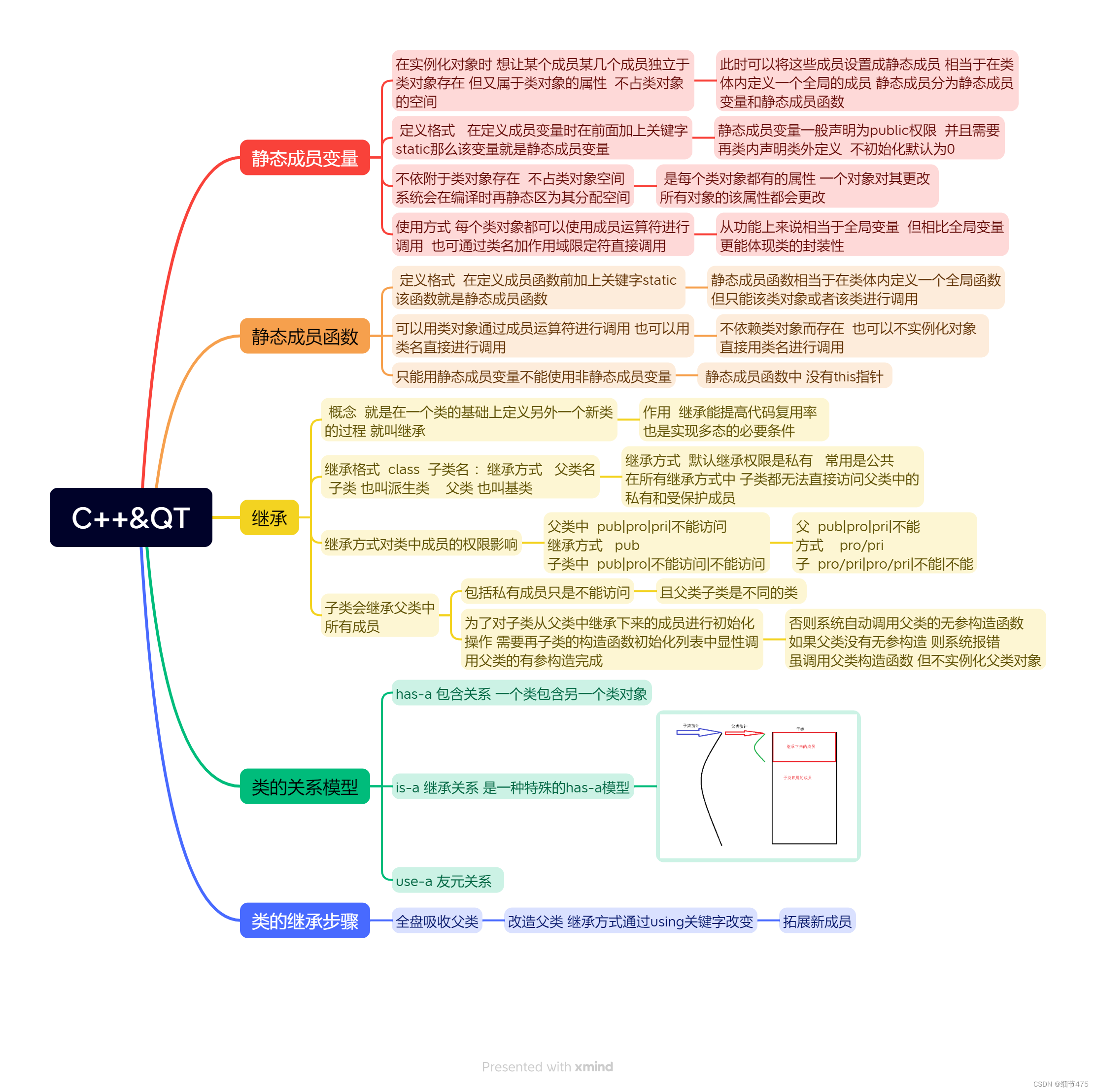
#include <iostream>
#define PI 3
using namespace std;
class Shape
{
protected:
int zc;
int mj;
public:
Shape(){}
Shape(int z,int m):zc(z),mj(z){}
~Shape(){}
Shape(const Shape &other):zc(other.zc),mj(other.mj)
{
cout<<"拷贝构造函数"<<endl;
}
Shape & operator=(const Shape &other)
{
this->zc = other.zc;
this->mj = other.mj;
cout<<"拷贝赋值函数"<<endl;
return *this;
}
};
class Circle:public Shape
{
private:
int bj;
public:
Circle(){}
Circle(int r):bj(r){}
~Circle(){}
Circle(const Circle &other):bj(other.bj){}
Circle &operator=(const Circle &other)
{
if(this != &other)
{
this->bj= other.bj;
}
cout<<"拷贝赋值函数"<<endl;
return *this;
}
int get_zc()//获取周长函数
{
this->zc=2*PI*bj;
return this->zc;
}
int get_mj()
{
this->mj=PI*bj*bj;
return this->mj;
}
};
class Rect:public Circle
{
private:
int cd;
int kd;
public:
Rect (){}
Rect (int c,int k):cd(c),kd(k){}
Rect & operator=(const Rect &other)
{
if(this != &other)
{
this->cd = other.cd;
this->kd = other.kd;
}
cout<<"拷贝赋值函数"<<endl;
return *this;
}
int get_zc()//获取周长函数
{
this->zc = 2*(cd+kd);
return this->zc;
}
int get_mj()//获取面积函数
{
this->mj = cd*kd;
return this->mj;
}
};
int main()
{
Circle c(2);
cout<<"c周长="<<c.get_zc()<<endl;
cout<<"c面积="<<c.get_mj()<<endl;
Circle c1;
c1=c;
cout<<"c1周长="<<c.get_zc()<<endl;
cout<<"c1面积="<<c.get_mj()<<endl;
Rect r(3,4);
cout<<"r周长="<<r.get_zc()<<endl;
cout<<"r面积="<<r.get_mj()<<endl;
Rect r1=r;
cout<<"r1周长="<<r.get_zc()<<endl;
cout<<"r1面积="<<r.get_mj()<<endl;
return 0;
}